Terminate called after throwing an instance of an exception, core dumped
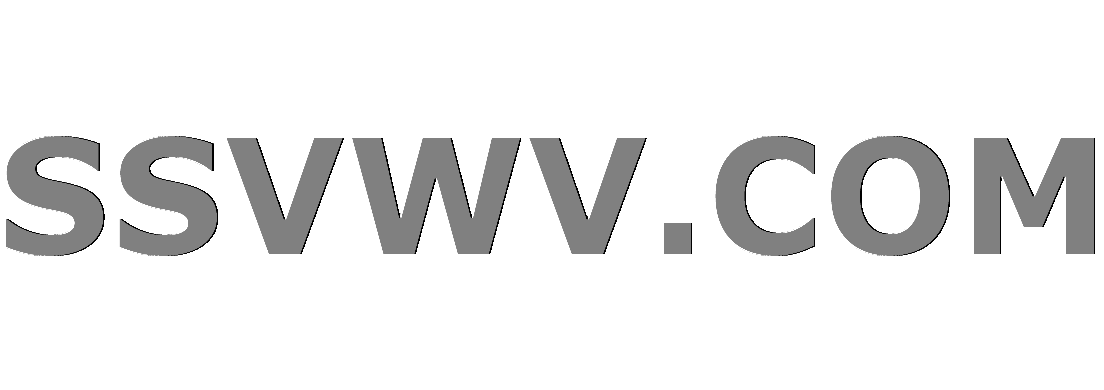
Multi tool use
I am going over C++ exceptions and am running into an error that I am unsure of why it is giving me issues:
#include <iostream>
#include <exception>
class err : public std::exception
{
public:
const char* what() const noexcept { return "error"; }
};
void f() throw()
{
throw err();
}
int main()
{
try
{
f();
}
catch (const err& e)
{
std::cout << e.what() << std::endl;
}
}
When I run it, I get the following runtime error:
terminate called after throwing an instance of 'err'
what(): error
Aborted (core dumped)
If I move the try/catch
logic completely to f()
, i.e.
void f()
{
try
{
throw err();
}
catch (const err& e)
{
std::cout << e.what() << std::endl;
}
}
And just call it from main
(without the try/catch block in main), then there is no error. Am I not understanding something, as it relates to throwing exceptions from functions?
c++ c++11 exception
add a comment |
I am going over C++ exceptions and am running into an error that I am unsure of why it is giving me issues:
#include <iostream>
#include <exception>
class err : public std::exception
{
public:
const char* what() const noexcept { return "error"; }
};
void f() throw()
{
throw err();
}
int main()
{
try
{
f();
}
catch (const err& e)
{
std::cout << e.what() << std::endl;
}
}
When I run it, I get the following runtime error:
terminate called after throwing an instance of 'err'
what(): error
Aborted (core dumped)
If I move the try/catch
logic completely to f()
, i.e.
void f()
{
try
{
throw err();
}
catch (const err& e)
{
std::cout << e.what() << std::endl;
}
}
And just call it from main
(without the try/catch block in main), then there is no error. Am I not understanding something, as it relates to throwing exceptions from functions?
c++ c++11 exception
9
void f() throw()
says that the function doesn't throw exceptions. And then you do.
– Neil Butterworth
Jan 2 at 18:42
2
Remove thethrow()
. You are saying "this function never throws" but then you violate that promise and throw anyway. That leads tostd::terminate
since you broke the rules of the language - at that point you cannot expect anything.
– Jesper Juhl
Jan 2 at 18:57
add a comment |
I am going over C++ exceptions and am running into an error that I am unsure of why it is giving me issues:
#include <iostream>
#include <exception>
class err : public std::exception
{
public:
const char* what() const noexcept { return "error"; }
};
void f() throw()
{
throw err();
}
int main()
{
try
{
f();
}
catch (const err& e)
{
std::cout << e.what() << std::endl;
}
}
When I run it, I get the following runtime error:
terminate called after throwing an instance of 'err'
what(): error
Aborted (core dumped)
If I move the try/catch
logic completely to f()
, i.e.
void f()
{
try
{
throw err();
}
catch (const err& e)
{
std::cout << e.what() << std::endl;
}
}
And just call it from main
(without the try/catch block in main), then there is no error. Am I not understanding something, as it relates to throwing exceptions from functions?
c++ c++11 exception
I am going over C++ exceptions and am running into an error that I am unsure of why it is giving me issues:
#include <iostream>
#include <exception>
class err : public std::exception
{
public:
const char* what() const noexcept { return "error"; }
};
void f() throw()
{
throw err();
}
int main()
{
try
{
f();
}
catch (const err& e)
{
std::cout << e.what() << std::endl;
}
}
When I run it, I get the following runtime error:
terminate called after throwing an instance of 'err'
what(): error
Aborted (core dumped)
If I move the try/catch
logic completely to f()
, i.e.
void f()
{
try
{
throw err();
}
catch (const err& e)
{
std::cout << e.what() << std::endl;
}
}
And just call it from main
(without the try/catch block in main), then there is no error. Am I not understanding something, as it relates to throwing exceptions from functions?
c++ c++11 exception
c++ c++11 exception
edited Jan 2 at 18:48


Ron
10.5k21834
10.5k21834
asked Jan 2 at 18:40
HectorJ
675
675
9
void f() throw()
says that the function doesn't throw exceptions. And then you do.
– Neil Butterworth
Jan 2 at 18:42
2
Remove thethrow()
. You are saying "this function never throws" but then you violate that promise and throw anyway. That leads tostd::terminate
since you broke the rules of the language - at that point you cannot expect anything.
– Jesper Juhl
Jan 2 at 18:57
add a comment |
9
void f() throw()
says that the function doesn't throw exceptions. And then you do.
– Neil Butterworth
Jan 2 at 18:42
2
Remove thethrow()
. You are saying "this function never throws" but then you violate that promise and throw anyway. That leads tostd::terminate
since you broke the rules of the language - at that point you cannot expect anything.
– Jesper Juhl
Jan 2 at 18:57
9
9
void f() throw()
says that the function doesn't throw exceptions. And then you do.– Neil Butterworth
Jan 2 at 18:42
void f() throw()
says that the function doesn't throw exceptions. And then you do.– Neil Butterworth
Jan 2 at 18:42
2
2
Remove the
throw()
. You are saying "this function never throws" but then you violate that promise and throw anyway. That leads to std::terminate
since you broke the rules of the language - at that point you cannot expect anything.– Jesper Juhl
Jan 2 at 18:57
Remove the
throw()
. You are saying "this function never throws" but then you violate that promise and throw anyway. That leads to std::terminate
since you broke the rules of the language - at that point you cannot expect anything.– Jesper Juhl
Jan 2 at 18:57
add a comment |
1 Answer
1
active
oldest
votes
The throw()
in void f() throw()
is a dynamic exception specification and is deprecated since c++11. It's was supposed to be used to list the exceptions a function could throw. An empty specification (throw()
) means there are no exceptions that your function could throw. Trying to throw an exception from such a function calls std::unexpected
which, by default, terminates.
Since c++11 the preferred way of specifying that a function cannot throw is to use noexcept
. For example void f() noexcept
.
Thank you! I had an incorrect understanding. It looks likenoexcept(false)
will provide the same functionality and looks to not have been deprecated
– HectorJ
Jan 2 at 18:59
4
@HectorJnoexcept(false)
is the default for most functions and usually does not need to be provided. I believe destructors arenoexcept(true)
by default and as far as I know that would be the only timenoexcept(false)
has meaning (though that's not recommended).
– François Andrieux
Jan 2 at 19:00
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54011523%2fterminate-called-after-throwing-an-instance-of-an-exception-core-dumped%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The throw()
in void f() throw()
is a dynamic exception specification and is deprecated since c++11. It's was supposed to be used to list the exceptions a function could throw. An empty specification (throw()
) means there are no exceptions that your function could throw. Trying to throw an exception from such a function calls std::unexpected
which, by default, terminates.
Since c++11 the preferred way of specifying that a function cannot throw is to use noexcept
. For example void f() noexcept
.
Thank you! I had an incorrect understanding. It looks likenoexcept(false)
will provide the same functionality and looks to not have been deprecated
– HectorJ
Jan 2 at 18:59
4
@HectorJnoexcept(false)
is the default for most functions and usually does not need to be provided. I believe destructors arenoexcept(true)
by default and as far as I know that would be the only timenoexcept(false)
has meaning (though that's not recommended).
– François Andrieux
Jan 2 at 19:00
add a comment |
The throw()
in void f() throw()
is a dynamic exception specification and is deprecated since c++11. It's was supposed to be used to list the exceptions a function could throw. An empty specification (throw()
) means there are no exceptions that your function could throw. Trying to throw an exception from such a function calls std::unexpected
which, by default, terminates.
Since c++11 the preferred way of specifying that a function cannot throw is to use noexcept
. For example void f() noexcept
.
Thank you! I had an incorrect understanding. It looks likenoexcept(false)
will provide the same functionality and looks to not have been deprecated
– HectorJ
Jan 2 at 18:59
4
@HectorJnoexcept(false)
is the default for most functions and usually does not need to be provided. I believe destructors arenoexcept(true)
by default and as far as I know that would be the only timenoexcept(false)
has meaning (though that's not recommended).
– François Andrieux
Jan 2 at 19:00
add a comment |
The throw()
in void f() throw()
is a dynamic exception specification and is deprecated since c++11. It's was supposed to be used to list the exceptions a function could throw. An empty specification (throw()
) means there are no exceptions that your function could throw. Trying to throw an exception from such a function calls std::unexpected
which, by default, terminates.
Since c++11 the preferred way of specifying that a function cannot throw is to use noexcept
. For example void f() noexcept
.
The throw()
in void f() throw()
is a dynamic exception specification and is deprecated since c++11. It's was supposed to be used to list the exceptions a function could throw. An empty specification (throw()
) means there are no exceptions that your function could throw. Trying to throw an exception from such a function calls std::unexpected
which, by default, terminates.
Since c++11 the preferred way of specifying that a function cannot throw is to use noexcept
. For example void f() noexcept
.
edited Jan 2 at 18:59
answered Jan 2 at 18:44


François Andrieux
15.4k32647
15.4k32647
Thank you! I had an incorrect understanding. It looks likenoexcept(false)
will provide the same functionality and looks to not have been deprecated
– HectorJ
Jan 2 at 18:59
4
@HectorJnoexcept(false)
is the default for most functions and usually does not need to be provided. I believe destructors arenoexcept(true)
by default and as far as I know that would be the only timenoexcept(false)
has meaning (though that's not recommended).
– François Andrieux
Jan 2 at 19:00
add a comment |
Thank you! I had an incorrect understanding. It looks likenoexcept(false)
will provide the same functionality and looks to not have been deprecated
– HectorJ
Jan 2 at 18:59
4
@HectorJnoexcept(false)
is the default for most functions and usually does not need to be provided. I believe destructors arenoexcept(true)
by default and as far as I know that would be the only timenoexcept(false)
has meaning (though that's not recommended).
– François Andrieux
Jan 2 at 19:00
Thank you! I had an incorrect understanding. It looks like
noexcept(false)
will provide the same functionality and looks to not have been deprecated– HectorJ
Jan 2 at 18:59
Thank you! I had an incorrect understanding. It looks like
noexcept(false)
will provide the same functionality and looks to not have been deprecated– HectorJ
Jan 2 at 18:59
4
4
@HectorJ
noexcept(false)
is the default for most functions and usually does not need to be provided. I believe destructors are noexcept(true)
by default and as far as I know that would be the only time noexcept(false)
has meaning (though that's not recommended).– François Andrieux
Jan 2 at 19:00
@HectorJ
noexcept(false)
is the default for most functions and usually does not need to be provided. I believe destructors are noexcept(true)
by default and as far as I know that would be the only time noexcept(false)
has meaning (though that's not recommended).– François Andrieux
Jan 2 at 19:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54011523%2fterminate-called-after-throwing-an-instance-of-an-exception-core-dumped%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
g,N4zu 3O6 UoKUx,YYk9LKMa0mHWtxkKOvwi5r HuDaGtAP4 t4Wjq0QogaGbnQWTh6ljZQ4P,FtPT
9
void f() throw()
says that the function doesn't throw exceptions. And then you do.– Neil Butterworth
Jan 2 at 18:42
2
Remove the
throw()
. You are saying "this function never throws" but then you violate that promise and throw anyway. That leads tostd::terminate
since you broke the rules of the language - at that point you cannot expect anything.– Jesper Juhl
Jan 2 at 18:57