Scheme function to duplicate every element of a list a specified number of times
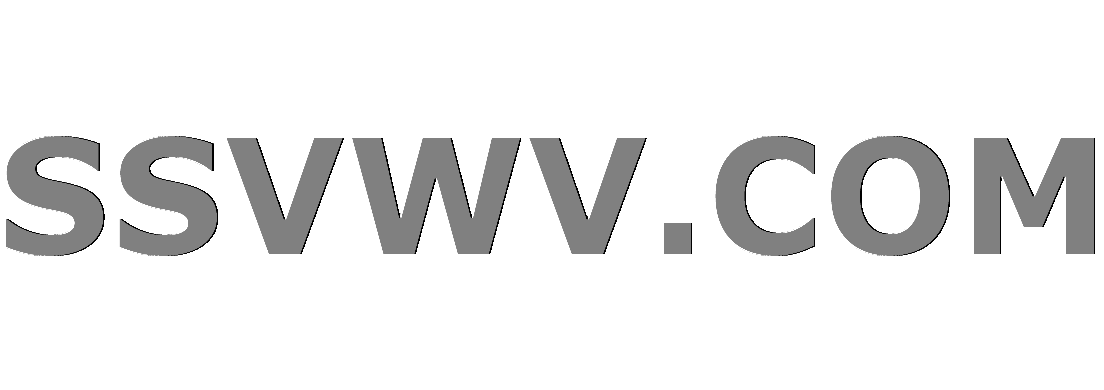
Multi tool use
up vote
1
down vote
favorite
This code takes a list and a integer and duplicates every element in the list the specified number of times.
(define (super-duper source count)
(define (next-super source left)
(if (zero? left)
(super-duper (cdr source) count)
(cons (super-duper (car source) count) (next-super source (- left 1)))))
(if (pair? source)
(cons (super-duper (car source) count) (next-super source (- count 1)))
source))
(display(super-duper '((x y) t) 3))
recursion scheme
add a comment |
up vote
1
down vote
favorite
This code takes a list and a integer and duplicates every element in the list the specified number of times.
(define (super-duper source count)
(define (next-super source left)
(if (zero? left)
(super-duper (cdr source) count)
(cons (super-duper (car source) count) (next-super source (- left 1)))))
(if (pair? source)
(cons (super-duper (car source) count) (next-super source (- count 1)))
source))
(display(super-duper '((x y) t) 3))
recursion scheme
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
This code takes a list and a integer and duplicates every element in the list the specified number of times.
(define (super-duper source count)
(define (next-super source left)
(if (zero? left)
(super-duper (cdr source) count)
(cons (super-duper (car source) count) (next-super source (- left 1)))))
(if (pair? source)
(cons (super-duper (car source) count) (next-super source (- count 1)))
source))
(display(super-duper '((x y) t) 3))
recursion scheme
This code takes a list and a integer and duplicates every element in the list the specified number of times.
(define (super-duper source count)
(define (next-super source left)
(if (zero? left)
(super-duper (cdr source) count)
(cons (super-duper (car source) count) (next-super source (- left 1)))))
(if (pair? source)
(cons (super-duper (car source) count) (next-super source (- count 1)))
source))
(display(super-duper '((x y) t) 3))
recursion scheme
recursion scheme
edited Sep 20 at 3:03


200_success
127k15148412
127k15148412
asked Sep 20 at 0:09
Dporth
182
182
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
I think you need to decide weather to repeat only on the first level of the list, or if you want to do a tree walk for lists inside lists. As is, this code produces a weird combination of both in an exponential explosion of the list, The following sample returns over 2 million leaf elements for an input of length 26 and a repeat of 10.
(leaf-count (super-duper '(a (b (c (d (e f g (h i) j k) l m n o) p q ) r (s t (u v) w ) x y) z) 10))
->> 2576420
(define (leaf-count Tree)
(cond ((null? Tree) 0)
((not (pair? Tree)) 1)
(else (+ (leaf-count (car Tree))
(leaf-count (cdr Tree))))))
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I think you need to decide weather to repeat only on the first level of the list, or if you want to do a tree walk for lists inside lists. As is, this code produces a weird combination of both in an exponential explosion of the list, The following sample returns over 2 million leaf elements for an input of length 26 and a repeat of 10.
(leaf-count (super-duper '(a (b (c (d (e f g (h i) j k) l m n o) p q ) r (s t (u v) w ) x y) z) 10))
->> 2576420
(define (leaf-count Tree)
(cond ((null? Tree) 0)
((not (pair? Tree)) 1)
(else (+ (leaf-count (car Tree))
(leaf-count (cdr Tree))))))
add a comment |
up vote
0
down vote
I think you need to decide weather to repeat only on the first level of the list, or if you want to do a tree walk for lists inside lists. As is, this code produces a weird combination of both in an exponential explosion of the list, The following sample returns over 2 million leaf elements for an input of length 26 and a repeat of 10.
(leaf-count (super-duper '(a (b (c (d (e f g (h i) j k) l m n o) p q ) r (s t (u v) w ) x y) z) 10))
->> 2576420
(define (leaf-count Tree)
(cond ((null? Tree) 0)
((not (pair? Tree)) 1)
(else (+ (leaf-count (car Tree))
(leaf-count (cdr Tree))))))
add a comment |
up vote
0
down vote
up vote
0
down vote
I think you need to decide weather to repeat only on the first level of the list, or if you want to do a tree walk for lists inside lists. As is, this code produces a weird combination of both in an exponential explosion of the list, The following sample returns over 2 million leaf elements for an input of length 26 and a repeat of 10.
(leaf-count (super-duper '(a (b (c (d (e f g (h i) j k) l m n o) p q ) r (s t (u v) w ) x y) z) 10))
->> 2576420
(define (leaf-count Tree)
(cond ((null? Tree) 0)
((not (pair? Tree)) 1)
(else (+ (leaf-count (car Tree))
(leaf-count (cdr Tree))))))
I think you need to decide weather to repeat only on the first level of the list, or if you want to do a tree walk for lists inside lists. As is, this code produces a weird combination of both in an exponential explosion of the list, The following sample returns over 2 million leaf elements for an input of length 26 and a repeat of 10.
(leaf-count (super-duper '(a (b (c (d (e f g (h i) j k) l m n o) p q ) r (s t (u v) w ) x y) z) 10))
->> 2576420
(define (leaf-count Tree)
(cond ((null? Tree) 0)
((not (pair? Tree)) 1)
(else (+ (leaf-count (car Tree))
(leaf-count (cdr Tree))))))
answered Sep 25 at 4:06
WorBlux
35116
35116
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f204022%2fscheme-function-to-duplicate-every-element-of-a-list-a-specified-number-of-times%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
64DSHLBTW,VYX,E rbJoN,ArWzAW5 s2O95RHQ,UEze6eX,VEOVYjMRgcjfgXGwSB7 Q2c,m7IngmYp9ZP,VopbdX1FE