Simple Rock, Paper, Scissors Python Game
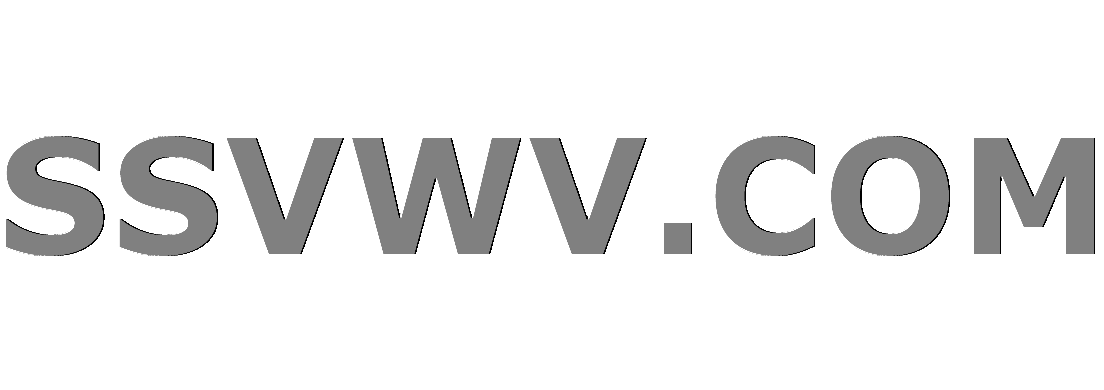
Multi tool use
$begingroup$
I tried to learn Python during the weekend and after getting some knowledge decided to make a small rock-paper-scissors game. I would be grateful for your input on how I could improve my code. All feedback is highly appreciated.
import random
def round_winner(choice):
ai_chosen = str(random.randint(1, 3))
print(f'AI chose {ai_chosen}')
if choice == '1' and ai_chosen == '2':
return 'ai'
elif choice == '2' and ai_chosen == '3':
return 'ai'
elif choice == '3' and ai_chosen == '1':
return 'ai'
elif choice == ai_chosen:
return 'tie'
else:
return 'player'
def display_round_winner(winner):
if winner == 'tie':
print('This round is tied!')
else:
print(f'The winner this round is the {winner.upper()}')
print(f'''
Current points as follows:
Player: {counter['player']}
AI: {counter['ai']}
Rounds Tied: {counter['tie']}
''')
def score_checker():
global game_ongoing
for key, value in counter.items():
if value == 2:
print(f'{key.upper()} wins the game!')
game_ongoing = False
def initializer():
global counter
message = '''
Please choose one of the following:
1: Rock
2: Paper
3: Scissors
'''
print(message)
choice_of_obj = input('What will it be: ')
if choice_of_obj in ['1', '2', '3']:
winner = round_winner(choice_of_obj)
counter[winner] += 1
display_round_winner(winner)
score_checker()
else:
print('Out of bounds')
counter = {
'player': 0,
'ai': 0,
'tie': 0
}
game_ongoing = True
while game_ongoing:
initializer()
python beginner rock-paper-scissors
New contributor
aleisley is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
I tried to learn Python during the weekend and after getting some knowledge decided to make a small rock-paper-scissors game. I would be grateful for your input on how I could improve my code. All feedback is highly appreciated.
import random
def round_winner(choice):
ai_chosen = str(random.randint(1, 3))
print(f'AI chose {ai_chosen}')
if choice == '1' and ai_chosen == '2':
return 'ai'
elif choice == '2' and ai_chosen == '3':
return 'ai'
elif choice == '3' and ai_chosen == '1':
return 'ai'
elif choice == ai_chosen:
return 'tie'
else:
return 'player'
def display_round_winner(winner):
if winner == 'tie':
print('This round is tied!')
else:
print(f'The winner this round is the {winner.upper()}')
print(f'''
Current points as follows:
Player: {counter['player']}
AI: {counter['ai']}
Rounds Tied: {counter['tie']}
''')
def score_checker():
global game_ongoing
for key, value in counter.items():
if value == 2:
print(f'{key.upper()} wins the game!')
game_ongoing = False
def initializer():
global counter
message = '''
Please choose one of the following:
1: Rock
2: Paper
3: Scissors
'''
print(message)
choice_of_obj = input('What will it be: ')
if choice_of_obj in ['1', '2', '3']:
winner = round_winner(choice_of_obj)
counter[winner] += 1
display_round_winner(winner)
score_checker()
else:
print('Out of bounds')
counter = {
'player': 0,
'ai': 0,
'tie': 0
}
game_ongoing = True
while game_ongoing:
initializer()
python beginner rock-paper-scissors
New contributor
aleisley is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
I tried to learn Python during the weekend and after getting some knowledge decided to make a small rock-paper-scissors game. I would be grateful for your input on how I could improve my code. All feedback is highly appreciated.
import random
def round_winner(choice):
ai_chosen = str(random.randint(1, 3))
print(f'AI chose {ai_chosen}')
if choice == '1' and ai_chosen == '2':
return 'ai'
elif choice == '2' and ai_chosen == '3':
return 'ai'
elif choice == '3' and ai_chosen == '1':
return 'ai'
elif choice == ai_chosen:
return 'tie'
else:
return 'player'
def display_round_winner(winner):
if winner == 'tie':
print('This round is tied!')
else:
print(f'The winner this round is the {winner.upper()}')
print(f'''
Current points as follows:
Player: {counter['player']}
AI: {counter['ai']}
Rounds Tied: {counter['tie']}
''')
def score_checker():
global game_ongoing
for key, value in counter.items():
if value == 2:
print(f'{key.upper()} wins the game!')
game_ongoing = False
def initializer():
global counter
message = '''
Please choose one of the following:
1: Rock
2: Paper
3: Scissors
'''
print(message)
choice_of_obj = input('What will it be: ')
if choice_of_obj in ['1', '2', '3']:
winner = round_winner(choice_of_obj)
counter[winner] += 1
display_round_winner(winner)
score_checker()
else:
print('Out of bounds')
counter = {
'player': 0,
'ai': 0,
'tie': 0
}
game_ongoing = True
while game_ongoing:
initializer()
python beginner rock-paper-scissors
New contributor
aleisley is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
I tried to learn Python during the weekend and after getting some knowledge decided to make a small rock-paper-scissors game. I would be grateful for your input on how I could improve my code. All feedback is highly appreciated.
import random
def round_winner(choice):
ai_chosen = str(random.randint(1, 3))
print(f'AI chose {ai_chosen}')
if choice == '1' and ai_chosen == '2':
return 'ai'
elif choice == '2' and ai_chosen == '3':
return 'ai'
elif choice == '3' and ai_chosen == '1':
return 'ai'
elif choice == ai_chosen:
return 'tie'
else:
return 'player'
def display_round_winner(winner):
if winner == 'tie':
print('This round is tied!')
else:
print(f'The winner this round is the {winner.upper()}')
print(f'''
Current points as follows:
Player: {counter['player']}
AI: {counter['ai']}
Rounds Tied: {counter['tie']}
''')
def score_checker():
global game_ongoing
for key, value in counter.items():
if value == 2:
print(f'{key.upper()} wins the game!')
game_ongoing = False
def initializer():
global counter
message = '''
Please choose one of the following:
1: Rock
2: Paper
3: Scissors
'''
print(message)
choice_of_obj = input('What will it be: ')
if choice_of_obj in ['1', '2', '3']:
winner = round_winner(choice_of_obj)
counter[winner] += 1
display_round_winner(winner)
score_checker()
else:
print('Out of bounds')
counter = {
'player': 0,
'ai': 0,
'tie': 0
}
game_ongoing = True
while game_ongoing:
initializer()
python beginner rock-paper-scissors
python beginner rock-paper-scissors
New contributor
aleisley is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
aleisley is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 1 hour ago


esote
2,7861938
2,7861938
New contributor
aleisley is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 1 hour ago
aleisleyaleisley
1
1
New contributor
aleisley is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
aleisley is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
aleisley is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
aleisley is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215714%2fsimple-rock-paper-scissors-python-game%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
aleisley is a new contributor. Be nice, and check out our Code of Conduct.
aleisley is a new contributor. Be nice, and check out our Code of Conduct.
aleisley is a new contributor. Be nice, and check out our Code of Conduct.
aleisley is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215714%2fsimple-rock-paper-scissors-python-game%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Zns qr0ru KrFFu9ltQ Id yFX1,uIdfzX,jSKM2IbTySq0cc4fHUJJmmxppbi,f,B