Unit testing for Splay Tree in Python
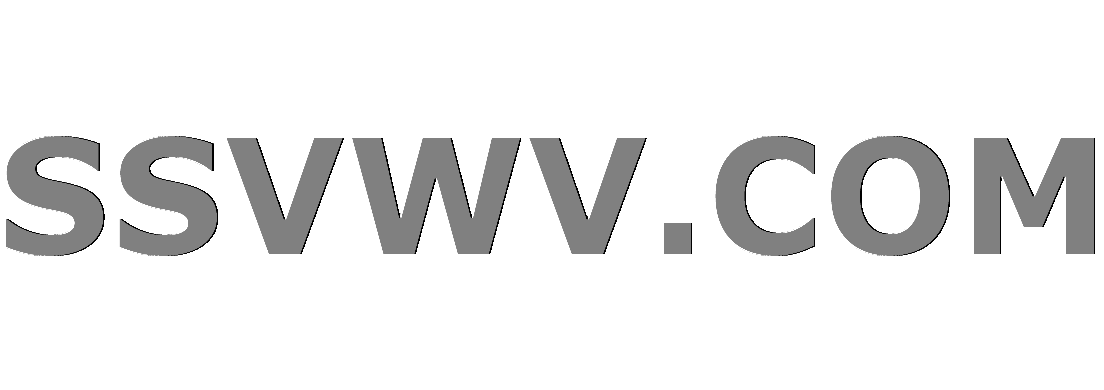
Multi tool use
I am creating a test class for the following code. splay_test.py which is the unit test code for splay tree. I needed help with writing unit tests for python to get %100 coverage. I am very bad at unit testing but have started off ok. just need someone to help me finish it off and maybe find any more bugs. My coverage for some reason isn't connecting the two files together so I can't see what my overall coverage is.
class Node:
def __init__(self, key):
self.key = key
self.left = self.right = None
def equals(self, node):
return self.key == node.key
class SplayTree:
def __init__(self):
self.root = None
self.header = Node(None) # For splay()
def insert(self, key):
if (self.root == None):
self.root = Node(key)
return #test
self.splay(key)
if self.root.key == key:
# If the key is already there in the tree, don't do anything.
return
n = Node(key)
if key < self.root.key:
n.left = self.root.left
n.right = self.root
self.root.left = None
else:
n.right = self.root.right
n.left = self.root
self.root.right = None
self.root = n
def remove(self, key):
self.splay(key)
if key != self.root.key:
raise 'key not found in tree' # do
# Now delete the root.
if self.root.left == None:
self.root = self.root.right
else:
x = self.root.right
self.root = self.root.left
self.splay(key)
self.root.right = x
def findMin(self):
if self.root == None:
return None
x = self.root
while x.left != None:
x = x.left
self.splay(x.key)
return x.key
def findMax(self):
if self.root == None:
return None
x = self.root
while (x.right != None):
x = x.right
self.splay(x.key)
return x.key
def find(self, key): # test
if self.root == None:
return None
self.splay(key)
if self.root.key != key:
return None
return self.root.key
def isEmpty(self):
return self.root == None
def splay(self, key): # test
l = r = self.header
t = self.root
self.header.left = self.header.right = None
while True:
if key < t.key:
if t.left == None:
break
if key < t.left.key:
y = t.left
t.left = y.right
y.right = t
t = y
if t.left == None:
break
r.left = t
r = t
t = t.left
elif key > t.key:
if t.right == None:
break
if key > t.right.key:
y = t.right
t.right = y.left
y.left = t
t = y
if t.right == None:
break
l.right = t
l = t
t = t.right
else:
break
l.right = t.left
r.left = t.right
t.left = self.header.right
t.right = self.header.left
self.root = t
What I have so far
import unittest
from splay import SplayTree
class TestCase(unittest.TestCase):
def setUp(self):
self.keys = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
self.t = SplayTree()
for key in self.keys:
self.t.insert(key)
def testInsert(self):
for key in self.keys:
self.assertEquals(key, self.t.find(key))
def testRemove(self):
for key in self.keys:
self.t.remove(key)
self.assertEquals(self.t.find(key), None)
def testLargeInserts(self):
t = SplayTree()
nums = 40000
gap = 307
i = gap
while i != 0:
t.insert(i)
i = (i + gap) % nums
def testIsEmpty(self):
self.assertFalse(self.t.isEmpty())
t = SplayTree()
self.assertTrue(t.isEmpty())
def testMinMax(self):
self.assertEquals(self.t.findMin(), 0)
self.assertEquals(self.t.findMax(), 9)
Tests I have done so far
import unittest
from splay import SplayTree
class TestCase(unittest.TestCase):
def setUp(self):
self.keys = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
self.t = SplayTree()
for key in self.keys:
self.t.insert(key)
def testInsert(self):
for key in self.keys:
self.assertEquals(key, self.t.find(key))
def testRemove(self):
for key in self.keys:
self.t.remove(key)
self.assertEquals(self.t.find(key), None)
def testLargeInserts(self):
t = SplayTree()
nums = 40000
gap = 307
i = gap
while i != 0:
t.insert(i)
i = (i + gap) % nums
def testIsEmpty(self):
self.assertFalse(self.t.isEmpty())
t = SplayTree()
self.assertTrue(t.isEmpty())
def testMinMax(self):
self.assertEquals(self.t.findMin(), 0)
self.assertEquals(self.t.findMax(), 9)
if __name__ == "__main__":
unittest.main()
python unit-testing tree
New contributor
Max is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
I am creating a test class for the following code. splay_test.py which is the unit test code for splay tree. I needed help with writing unit tests for python to get %100 coverage. I am very bad at unit testing but have started off ok. just need someone to help me finish it off and maybe find any more bugs. My coverage for some reason isn't connecting the two files together so I can't see what my overall coverage is.
class Node:
def __init__(self, key):
self.key = key
self.left = self.right = None
def equals(self, node):
return self.key == node.key
class SplayTree:
def __init__(self):
self.root = None
self.header = Node(None) # For splay()
def insert(self, key):
if (self.root == None):
self.root = Node(key)
return #test
self.splay(key)
if self.root.key == key:
# If the key is already there in the tree, don't do anything.
return
n = Node(key)
if key < self.root.key:
n.left = self.root.left
n.right = self.root
self.root.left = None
else:
n.right = self.root.right
n.left = self.root
self.root.right = None
self.root = n
def remove(self, key):
self.splay(key)
if key != self.root.key:
raise 'key not found in tree' # do
# Now delete the root.
if self.root.left == None:
self.root = self.root.right
else:
x = self.root.right
self.root = self.root.left
self.splay(key)
self.root.right = x
def findMin(self):
if self.root == None:
return None
x = self.root
while x.left != None:
x = x.left
self.splay(x.key)
return x.key
def findMax(self):
if self.root == None:
return None
x = self.root
while (x.right != None):
x = x.right
self.splay(x.key)
return x.key
def find(self, key): # test
if self.root == None:
return None
self.splay(key)
if self.root.key != key:
return None
return self.root.key
def isEmpty(self):
return self.root == None
def splay(self, key): # test
l = r = self.header
t = self.root
self.header.left = self.header.right = None
while True:
if key < t.key:
if t.left == None:
break
if key < t.left.key:
y = t.left
t.left = y.right
y.right = t
t = y
if t.left == None:
break
r.left = t
r = t
t = t.left
elif key > t.key:
if t.right == None:
break
if key > t.right.key:
y = t.right
t.right = y.left
y.left = t
t = y
if t.right == None:
break
l.right = t
l = t
t = t.right
else:
break
l.right = t.left
r.left = t.right
t.left = self.header.right
t.right = self.header.left
self.root = t
What I have so far
import unittest
from splay import SplayTree
class TestCase(unittest.TestCase):
def setUp(self):
self.keys = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
self.t = SplayTree()
for key in self.keys:
self.t.insert(key)
def testInsert(self):
for key in self.keys:
self.assertEquals(key, self.t.find(key))
def testRemove(self):
for key in self.keys:
self.t.remove(key)
self.assertEquals(self.t.find(key), None)
def testLargeInserts(self):
t = SplayTree()
nums = 40000
gap = 307
i = gap
while i != 0:
t.insert(i)
i = (i + gap) % nums
def testIsEmpty(self):
self.assertFalse(self.t.isEmpty())
t = SplayTree()
self.assertTrue(t.isEmpty())
def testMinMax(self):
self.assertEquals(self.t.findMin(), 0)
self.assertEquals(self.t.findMax(), 9)
Tests I have done so far
import unittest
from splay import SplayTree
class TestCase(unittest.TestCase):
def setUp(self):
self.keys = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
self.t = SplayTree()
for key in self.keys:
self.t.insert(key)
def testInsert(self):
for key in self.keys:
self.assertEquals(key, self.t.find(key))
def testRemove(self):
for key in self.keys:
self.t.remove(key)
self.assertEquals(self.t.find(key), None)
def testLargeInserts(self):
t = SplayTree()
nums = 40000
gap = 307
i = gap
while i != 0:
t.insert(i)
i = (i + gap) % nums
def testIsEmpty(self):
self.assertFalse(self.t.isEmpty())
t = SplayTree()
self.assertTrue(t.isEmpty())
def testMinMax(self):
self.assertEquals(self.t.findMin(), 0)
self.assertEquals(self.t.findMax(), 9)
if __name__ == "__main__":
unittest.main()
python unit-testing tree
New contributor
Max is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Rather than writing anequals
method, consider using__eq__
; do some reading here: docs.python.org/3/reference/datamodel.html#object.__eq__
– Reinderien
Dec 18 at 15:25
add a comment |
I am creating a test class for the following code. splay_test.py which is the unit test code for splay tree. I needed help with writing unit tests for python to get %100 coverage. I am very bad at unit testing but have started off ok. just need someone to help me finish it off and maybe find any more bugs. My coverage for some reason isn't connecting the two files together so I can't see what my overall coverage is.
class Node:
def __init__(self, key):
self.key = key
self.left = self.right = None
def equals(self, node):
return self.key == node.key
class SplayTree:
def __init__(self):
self.root = None
self.header = Node(None) # For splay()
def insert(self, key):
if (self.root == None):
self.root = Node(key)
return #test
self.splay(key)
if self.root.key == key:
# If the key is already there in the tree, don't do anything.
return
n = Node(key)
if key < self.root.key:
n.left = self.root.left
n.right = self.root
self.root.left = None
else:
n.right = self.root.right
n.left = self.root
self.root.right = None
self.root = n
def remove(self, key):
self.splay(key)
if key != self.root.key:
raise 'key not found in tree' # do
# Now delete the root.
if self.root.left == None:
self.root = self.root.right
else:
x = self.root.right
self.root = self.root.left
self.splay(key)
self.root.right = x
def findMin(self):
if self.root == None:
return None
x = self.root
while x.left != None:
x = x.left
self.splay(x.key)
return x.key
def findMax(self):
if self.root == None:
return None
x = self.root
while (x.right != None):
x = x.right
self.splay(x.key)
return x.key
def find(self, key): # test
if self.root == None:
return None
self.splay(key)
if self.root.key != key:
return None
return self.root.key
def isEmpty(self):
return self.root == None
def splay(self, key): # test
l = r = self.header
t = self.root
self.header.left = self.header.right = None
while True:
if key < t.key:
if t.left == None:
break
if key < t.left.key:
y = t.left
t.left = y.right
y.right = t
t = y
if t.left == None:
break
r.left = t
r = t
t = t.left
elif key > t.key:
if t.right == None:
break
if key > t.right.key:
y = t.right
t.right = y.left
y.left = t
t = y
if t.right == None:
break
l.right = t
l = t
t = t.right
else:
break
l.right = t.left
r.left = t.right
t.left = self.header.right
t.right = self.header.left
self.root = t
What I have so far
import unittest
from splay import SplayTree
class TestCase(unittest.TestCase):
def setUp(self):
self.keys = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
self.t = SplayTree()
for key in self.keys:
self.t.insert(key)
def testInsert(self):
for key in self.keys:
self.assertEquals(key, self.t.find(key))
def testRemove(self):
for key in self.keys:
self.t.remove(key)
self.assertEquals(self.t.find(key), None)
def testLargeInserts(self):
t = SplayTree()
nums = 40000
gap = 307
i = gap
while i != 0:
t.insert(i)
i = (i + gap) % nums
def testIsEmpty(self):
self.assertFalse(self.t.isEmpty())
t = SplayTree()
self.assertTrue(t.isEmpty())
def testMinMax(self):
self.assertEquals(self.t.findMin(), 0)
self.assertEquals(self.t.findMax(), 9)
Tests I have done so far
import unittest
from splay import SplayTree
class TestCase(unittest.TestCase):
def setUp(self):
self.keys = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
self.t = SplayTree()
for key in self.keys:
self.t.insert(key)
def testInsert(self):
for key in self.keys:
self.assertEquals(key, self.t.find(key))
def testRemove(self):
for key in self.keys:
self.t.remove(key)
self.assertEquals(self.t.find(key), None)
def testLargeInserts(self):
t = SplayTree()
nums = 40000
gap = 307
i = gap
while i != 0:
t.insert(i)
i = (i + gap) % nums
def testIsEmpty(self):
self.assertFalse(self.t.isEmpty())
t = SplayTree()
self.assertTrue(t.isEmpty())
def testMinMax(self):
self.assertEquals(self.t.findMin(), 0)
self.assertEquals(self.t.findMax(), 9)
if __name__ == "__main__":
unittest.main()
python unit-testing tree
New contributor
Max is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I am creating a test class for the following code. splay_test.py which is the unit test code for splay tree. I needed help with writing unit tests for python to get %100 coverage. I am very bad at unit testing but have started off ok. just need someone to help me finish it off and maybe find any more bugs. My coverage for some reason isn't connecting the two files together so I can't see what my overall coverage is.
class Node:
def __init__(self, key):
self.key = key
self.left = self.right = None
def equals(self, node):
return self.key == node.key
class SplayTree:
def __init__(self):
self.root = None
self.header = Node(None) # For splay()
def insert(self, key):
if (self.root == None):
self.root = Node(key)
return #test
self.splay(key)
if self.root.key == key:
# If the key is already there in the tree, don't do anything.
return
n = Node(key)
if key < self.root.key:
n.left = self.root.left
n.right = self.root
self.root.left = None
else:
n.right = self.root.right
n.left = self.root
self.root.right = None
self.root = n
def remove(self, key):
self.splay(key)
if key != self.root.key:
raise 'key not found in tree' # do
# Now delete the root.
if self.root.left == None:
self.root = self.root.right
else:
x = self.root.right
self.root = self.root.left
self.splay(key)
self.root.right = x
def findMin(self):
if self.root == None:
return None
x = self.root
while x.left != None:
x = x.left
self.splay(x.key)
return x.key
def findMax(self):
if self.root == None:
return None
x = self.root
while (x.right != None):
x = x.right
self.splay(x.key)
return x.key
def find(self, key): # test
if self.root == None:
return None
self.splay(key)
if self.root.key != key:
return None
return self.root.key
def isEmpty(self):
return self.root == None
def splay(self, key): # test
l = r = self.header
t = self.root
self.header.left = self.header.right = None
while True:
if key < t.key:
if t.left == None:
break
if key < t.left.key:
y = t.left
t.left = y.right
y.right = t
t = y
if t.left == None:
break
r.left = t
r = t
t = t.left
elif key > t.key:
if t.right == None:
break
if key > t.right.key:
y = t.right
t.right = y.left
y.left = t
t = y
if t.right == None:
break
l.right = t
l = t
t = t.right
else:
break
l.right = t.left
r.left = t.right
t.left = self.header.right
t.right = self.header.left
self.root = t
What I have so far
import unittest
from splay import SplayTree
class TestCase(unittest.TestCase):
def setUp(self):
self.keys = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
self.t = SplayTree()
for key in self.keys:
self.t.insert(key)
def testInsert(self):
for key in self.keys:
self.assertEquals(key, self.t.find(key))
def testRemove(self):
for key in self.keys:
self.t.remove(key)
self.assertEquals(self.t.find(key), None)
def testLargeInserts(self):
t = SplayTree()
nums = 40000
gap = 307
i = gap
while i != 0:
t.insert(i)
i = (i + gap) % nums
def testIsEmpty(self):
self.assertFalse(self.t.isEmpty())
t = SplayTree()
self.assertTrue(t.isEmpty())
def testMinMax(self):
self.assertEquals(self.t.findMin(), 0)
self.assertEquals(self.t.findMax(), 9)
Tests I have done so far
import unittest
from splay import SplayTree
class TestCase(unittest.TestCase):
def setUp(self):
self.keys = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
self.t = SplayTree()
for key in self.keys:
self.t.insert(key)
def testInsert(self):
for key in self.keys:
self.assertEquals(key, self.t.find(key))
def testRemove(self):
for key in self.keys:
self.t.remove(key)
self.assertEquals(self.t.find(key), None)
def testLargeInserts(self):
t = SplayTree()
nums = 40000
gap = 307
i = gap
while i != 0:
t.insert(i)
i = (i + gap) % nums
def testIsEmpty(self):
self.assertFalse(self.t.isEmpty())
t = SplayTree()
self.assertTrue(t.isEmpty())
def testMinMax(self):
self.assertEquals(self.t.findMin(), 0)
self.assertEquals(self.t.findMax(), 9)
if __name__ == "__main__":
unittest.main()
python unit-testing tree
python unit-testing tree
New contributor
Max is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Max is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Dec 18 at 13:44
Graipher
23.4k53485
23.4k53485
New contributor
Max is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Dec 18 at 13:05
Max
311
311
New contributor
Max is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Max is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Max is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Rather than writing anequals
method, consider using__eq__
; do some reading here: docs.python.org/3/reference/datamodel.html#object.__eq__
– Reinderien
Dec 18 at 15:25
add a comment |
Rather than writing anequals
method, consider using__eq__
; do some reading here: docs.python.org/3/reference/datamodel.html#object.__eq__
– Reinderien
Dec 18 at 15:25
Rather than writing an
equals
method, consider using __eq__
; do some reading here: docs.python.org/3/reference/datamodel.html#object.__eq__– Reinderien
Dec 18 at 15:25
Rather than writing an
equals
method, consider using __eq__
; do some reading here: docs.python.org/3/reference/datamodel.html#object.__eq__– Reinderien
Dec 18 at 15:25
add a comment |
1 Answer
1
active
oldest
votes
You've already done a pretty good job adding these tests and covering a big chunk of the code under test.
The problem is, if we look at the branch coverage, we'll see that quite a few of the branches are not reached as the implementation is relatively "complex" - by code complexity standards:
python -m pytest test_tree.py --cov-branch --cov=tree --cov-report html
Using pytest
and pytest-cov
plugin, tree
here is the module/package name for which to measure coverage.
Other observations:
self.assertEquals()
is deprecated in favor ofself.assertEqual()
- even though
setUp
andtearDown
are the legacy camel-case Junit-inspired names, consider following PEP8 and naming your methods in an lower_case_with_underscores style
- look into defining your tree as a
pytest
fixture
- look into property-based-testing with Hypothesis as a means to generate possible input nodes for your tree
- focus on the more complex parts first as this is where the possibility of having a problem is higher - the
splay()
method should probably be tested separately and directly checking all the major variations of re-balancing the tree when a new node is added
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Max is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f209904%2funit-testing-for-splay-tree-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You've already done a pretty good job adding these tests and covering a big chunk of the code under test.
The problem is, if we look at the branch coverage, we'll see that quite a few of the branches are not reached as the implementation is relatively "complex" - by code complexity standards:
python -m pytest test_tree.py --cov-branch --cov=tree --cov-report html
Using pytest
and pytest-cov
plugin, tree
here is the module/package name for which to measure coverage.
Other observations:
self.assertEquals()
is deprecated in favor ofself.assertEqual()
- even though
setUp
andtearDown
are the legacy camel-case Junit-inspired names, consider following PEP8 and naming your methods in an lower_case_with_underscores style
- look into defining your tree as a
pytest
fixture
- look into property-based-testing with Hypothesis as a means to generate possible input nodes for your tree
- focus on the more complex parts first as this is where the possibility of having a problem is higher - the
splay()
method should probably be tested separately and directly checking all the major variations of re-balancing the tree when a new node is added
add a comment |
You've already done a pretty good job adding these tests and covering a big chunk of the code under test.
The problem is, if we look at the branch coverage, we'll see that quite a few of the branches are not reached as the implementation is relatively "complex" - by code complexity standards:
python -m pytest test_tree.py --cov-branch --cov=tree --cov-report html
Using pytest
and pytest-cov
plugin, tree
here is the module/package name for which to measure coverage.
Other observations:
self.assertEquals()
is deprecated in favor ofself.assertEqual()
- even though
setUp
andtearDown
are the legacy camel-case Junit-inspired names, consider following PEP8 and naming your methods in an lower_case_with_underscores style
- look into defining your tree as a
pytest
fixture
- look into property-based-testing with Hypothesis as a means to generate possible input nodes for your tree
- focus on the more complex parts first as this is where the possibility of having a problem is higher - the
splay()
method should probably be tested separately and directly checking all the major variations of re-balancing the tree when a new node is added
add a comment |
You've already done a pretty good job adding these tests and covering a big chunk of the code under test.
The problem is, if we look at the branch coverage, we'll see that quite a few of the branches are not reached as the implementation is relatively "complex" - by code complexity standards:
python -m pytest test_tree.py --cov-branch --cov=tree --cov-report html
Using pytest
and pytest-cov
plugin, tree
here is the module/package name for which to measure coverage.
Other observations:
self.assertEquals()
is deprecated in favor ofself.assertEqual()
- even though
setUp
andtearDown
are the legacy camel-case Junit-inspired names, consider following PEP8 and naming your methods in an lower_case_with_underscores style
- look into defining your tree as a
pytest
fixture
- look into property-based-testing with Hypothesis as a means to generate possible input nodes for your tree
- focus on the more complex parts first as this is where the possibility of having a problem is higher - the
splay()
method should probably be tested separately and directly checking all the major variations of re-balancing the tree when a new node is added
You've already done a pretty good job adding these tests and covering a big chunk of the code under test.
The problem is, if we look at the branch coverage, we'll see that quite a few of the branches are not reached as the implementation is relatively "complex" - by code complexity standards:
python -m pytest test_tree.py --cov-branch --cov=tree --cov-report html
Using pytest
and pytest-cov
plugin, tree
here is the module/package name for which to measure coverage.
Other observations:
self.assertEquals()
is deprecated in favor ofself.assertEqual()
- even though
setUp
andtearDown
are the legacy camel-case Junit-inspired names, consider following PEP8 and naming your methods in an lower_case_with_underscores style
- look into defining your tree as a
pytest
fixture
- look into property-based-testing with Hypothesis as a means to generate possible input nodes for your tree
- focus on the more complex parts first as this is where the possibility of having a problem is higher - the
splay()
method should probably be tested separately and directly checking all the major variations of re-balancing the tree when a new node is added
edited Dec 18 at 16:21
answered Dec 18 at 16:15


alecxe
14.9k53478
14.9k53478
add a comment |
add a comment |
Max is a new contributor. Be nice, and check out our Code of Conduct.
Max is a new contributor. Be nice, and check out our Code of Conduct.
Max is a new contributor. Be nice, and check out our Code of Conduct.
Max is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f209904%2funit-testing-for-splay-tree-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lZd8 WI XG,F42yw Ap9eF29qbhto7h yGy,D6nk615t6Ahved3RaWE9
Rather than writing an
equals
method, consider using__eq__
; do some reading here: docs.python.org/3/reference/datamodel.html#object.__eq__– Reinderien
Dec 18 at 15:25