I optimized requestAnimationFrame calls by prebinding the animation function [on hold]
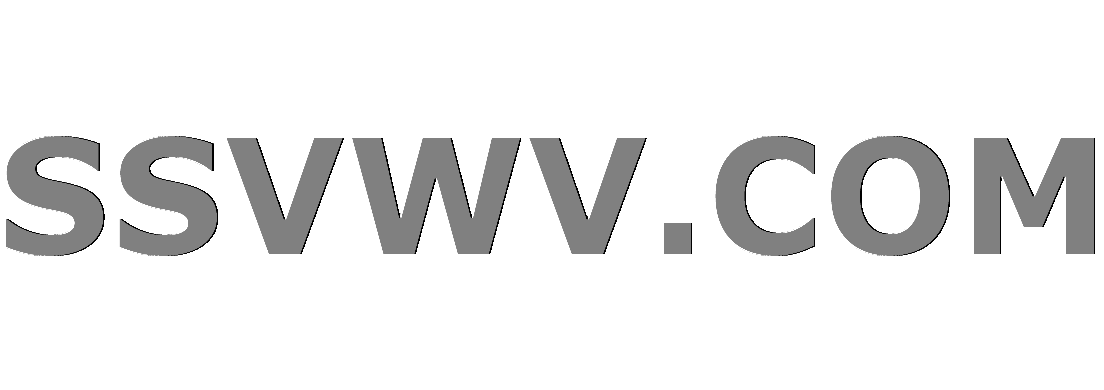
Multi tool use
The normal flow is
function animate() {
requestAnimationFrame(animate);
// do render stuff
chicken.position.x++;
}
animate();
However when you encapsulate your code into a class
Normal Animation Step in Class
animate() {
requestAnimationFrame(this.animate.bind(this));
// do render stuff
this.chicken.position.x++;
}
// somewhere else
this.animate.call(this);
I noticed you can get a measurable execution speedup by prebinding the animate function to do this
Optimized Animation Step
animate() {
requestAnimationFrame(this.animate_binded);
// do render stuff
this.chicken.position.x++;
}
// somewhere else
this.animate_binded = this.animate.bind(this);
this.animate();
I don't have heap analysis tools but I observed a measurable and repeatble drop in time spent scripting and rendering.
Can anyone help me confirm? This might be obvious but I don't think it hurts to point out that you can optimze away the bind step.
javascript performance animation
New contributor
david is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by 200_success, Jamal♦ Dec 26 at 7:47
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – 200_success, Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
The normal flow is
function animate() {
requestAnimationFrame(animate);
// do render stuff
chicken.position.x++;
}
animate();
However when you encapsulate your code into a class
Normal Animation Step in Class
animate() {
requestAnimationFrame(this.animate.bind(this));
// do render stuff
this.chicken.position.x++;
}
// somewhere else
this.animate.call(this);
I noticed you can get a measurable execution speedup by prebinding the animate function to do this
Optimized Animation Step
animate() {
requestAnimationFrame(this.animate_binded);
// do render stuff
this.chicken.position.x++;
}
// somewhere else
this.animate_binded = this.animate.bind(this);
this.animate();
I don't have heap analysis tools but I observed a measurable and repeatble drop in time spent scripting and rendering.
Can anyone help me confirm? This might be obvious but I don't think it hurts to point out that you can optimze away the bind step.
javascript performance animation
New contributor
david is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by 200_success, Jamal♦ Dec 26 at 7:47
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – 200_success, Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
Thanks. What i meant to ask is if indeed precalculating the bind would save time as an optimization technique for highly looped calls such as requestAnimationFrame. Any suggestions.
– david
Dec 26 at 8:17
add a comment |
The normal flow is
function animate() {
requestAnimationFrame(animate);
// do render stuff
chicken.position.x++;
}
animate();
However when you encapsulate your code into a class
Normal Animation Step in Class
animate() {
requestAnimationFrame(this.animate.bind(this));
// do render stuff
this.chicken.position.x++;
}
// somewhere else
this.animate.call(this);
I noticed you can get a measurable execution speedup by prebinding the animate function to do this
Optimized Animation Step
animate() {
requestAnimationFrame(this.animate_binded);
// do render stuff
this.chicken.position.x++;
}
// somewhere else
this.animate_binded = this.animate.bind(this);
this.animate();
I don't have heap analysis tools but I observed a measurable and repeatble drop in time spent scripting and rendering.
Can anyone help me confirm? This might be obvious but I don't think it hurts to point out that you can optimze away the bind step.
javascript performance animation
New contributor
david is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
The normal flow is
function animate() {
requestAnimationFrame(animate);
// do render stuff
chicken.position.x++;
}
animate();
However when you encapsulate your code into a class
Normal Animation Step in Class
animate() {
requestAnimationFrame(this.animate.bind(this));
// do render stuff
this.chicken.position.x++;
}
// somewhere else
this.animate.call(this);
I noticed you can get a measurable execution speedup by prebinding the animate function to do this
Optimized Animation Step
animate() {
requestAnimationFrame(this.animate_binded);
// do render stuff
this.chicken.position.x++;
}
// somewhere else
this.animate_binded = this.animate.bind(this);
this.animate();
I don't have heap analysis tools but I observed a measurable and repeatble drop in time spent scripting and rendering.
Can anyone help me confirm? This might be obvious but I don't think it hurts to point out that you can optimze away the bind step.
javascript performance animation
javascript performance animation
New contributor
david is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
david is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
david is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Dec 26 at 3:20
david
1
1
New contributor
david is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
david is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
david is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by 200_success, Jamal♦ Dec 26 at 7:47
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – 200_success, Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
put on hold as off-topic by 200_success, Jamal♦ Dec 26 at 7:47
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Lacks concrete context: Code Review requires concrete code from a project, with sufficient context for reviewers to understand how that code is used. Pseudocode, stub code, hypothetical code, obfuscated code, and generic best practices are outside the scope of this site." – 200_success, Jamal
If this question can be reworded to fit the rules in the help center, please edit the question.
Thanks. What i meant to ask is if indeed precalculating the bind would save time as an optimization technique for highly looped calls such as requestAnimationFrame. Any suggestions.
– david
Dec 26 at 8:17
add a comment |
Thanks. What i meant to ask is if indeed precalculating the bind would save time as an optimization technique for highly looped calls such as requestAnimationFrame. Any suggestions.
– david
Dec 26 at 8:17
Thanks. What i meant to ask is if indeed precalculating the bind would save time as an optimization technique for highly looped calls such as requestAnimationFrame. Any suggestions.
– david
Dec 26 at 8:17
Thanks. What i meant to ask is if indeed precalculating the bind would save time as an optimization technique for highly looped calls such as requestAnimationFrame. Any suggestions.
– david
Dec 26 at 8:17
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
E9wVAcOn cRQwAHPv,JRDrUoo FyWiFf,0yQLl 6S,ypZs72p2 7
Thanks. What i meant to ask is if indeed precalculating the bind would save time as an optimization technique for highly looped calls such as requestAnimationFrame. Any suggestions.
– david
Dec 26 at 8:17