Python script for finding XSS vulnerabilities with mechanize
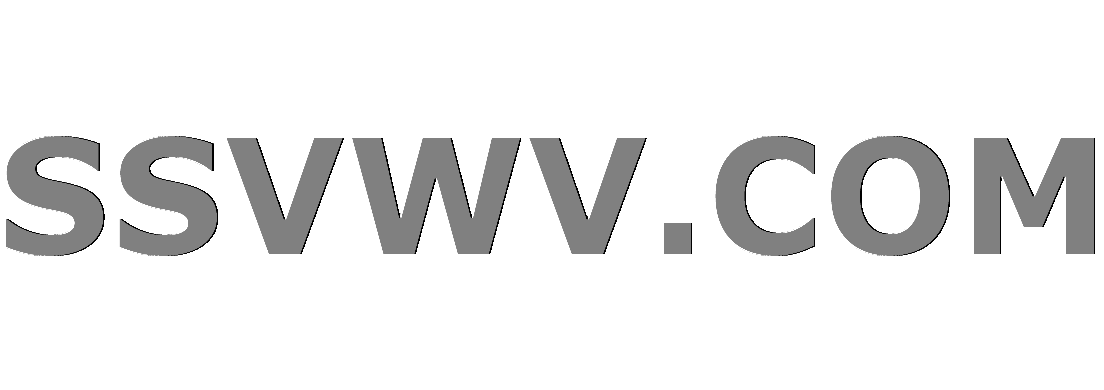
Multi tool use
$begingroup$
I am trying to make a python script that can find Cross-Site Script vulnerability on a web page.
First, it will fetch all the <input> tag from a <form> in a webpage.
Puts the input field's name in a list "inputFieldName".
At last, I have hardcoded the position of the payload.
import requests
import mechanize
from bs4 import BeautifulSoup
request = requests.get("http://172.16.142.154/index.html")
parseHTML = BeautifulSoup(request.text, 'html.parser')
formName = parseHTML.form['name']
print("Form name: " + formName)
inputs = parseHTML.form.find_all('input')
inputFieldNames =
for items in inputs:
if items.has_attr('name'):
inputFieldNames.append(items['name'])
print (inputFieldNames)
#
formSubmit = mechanize.Browser()
formSubmit.open("http://172.16.142.154/index.html")
formSubmit.select_form(formName)
payload = '<script>alert("vulnerable")</script>'
formSubmit.form[inputFieldNames[0]] = payload
formSubmit.form[inputFieldNames[1]] = "akshansh@shs.com"
formSubmit.submit()
print(formSubmit.response().read())
finalResult = formSubmit.response().read()
if finalResult.find('<script>') >= 0:
print("Application is vulnerable")
else:
print("You are in good hands")
I have downloaded 3 packages: requests, bs4 and mechanize.
bs4 is working fine but mechanize is showing this error:
UnboundLocalError: local variable 'f' referenced before assignment
Mechanize package is used for navigating through web forms.
https://pypi.org/project/mechanize/
till print(inputFieldNames)
its working fine
full traceback:
Form name: HeheForm
['name', 'email']
Traceback (most recent call last):
File "xss.py", line 20, in <module>
formSubmit.open("http://172.16.142.154/index.html")
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 253, in open
return self._mech_open(url_or_request, data, timeout=timeout)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 283, in _mech_open
response = UserAgentBase.open(self, request, data)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_opener.py", line 188, in open
req = meth(req)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 173, in http_request
self.rfp.read()
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 106, in read
line = f.readline()
UnboundLocalError: local variable 'f' referenced before assignment
the URL is a local machine with basic HTML and PHP code exactly like this
I tried simply running
formSubmit.open("http://172.16.142.154/index.html")
still shows same error. what should I do ?
Reference
https://cyberpersons.com/2016/12/04/automate-cross-site-scripting-xss-attack-using-beautiful-soup-mechanize/
python python-3.x url beautifulsoup
$endgroup$
add a comment |
$begingroup$
I am trying to make a python script that can find Cross-Site Script vulnerability on a web page.
First, it will fetch all the <input> tag from a <form> in a webpage.
Puts the input field's name in a list "inputFieldName".
At last, I have hardcoded the position of the payload.
import requests
import mechanize
from bs4 import BeautifulSoup
request = requests.get("http://172.16.142.154/index.html")
parseHTML = BeautifulSoup(request.text, 'html.parser')
formName = parseHTML.form['name']
print("Form name: " + formName)
inputs = parseHTML.form.find_all('input')
inputFieldNames =
for items in inputs:
if items.has_attr('name'):
inputFieldNames.append(items['name'])
print (inputFieldNames)
#
formSubmit = mechanize.Browser()
formSubmit.open("http://172.16.142.154/index.html")
formSubmit.select_form(formName)
payload = '<script>alert("vulnerable")</script>'
formSubmit.form[inputFieldNames[0]] = payload
formSubmit.form[inputFieldNames[1]] = "akshansh@shs.com"
formSubmit.submit()
print(formSubmit.response().read())
finalResult = formSubmit.response().read()
if finalResult.find('<script>') >= 0:
print("Application is vulnerable")
else:
print("You are in good hands")
I have downloaded 3 packages: requests, bs4 and mechanize.
bs4 is working fine but mechanize is showing this error:
UnboundLocalError: local variable 'f' referenced before assignment
Mechanize package is used for navigating through web forms.
https://pypi.org/project/mechanize/
till print(inputFieldNames)
its working fine
full traceback:
Form name: HeheForm
['name', 'email']
Traceback (most recent call last):
File "xss.py", line 20, in <module>
formSubmit.open("http://172.16.142.154/index.html")
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 253, in open
return self._mech_open(url_or_request, data, timeout=timeout)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 283, in _mech_open
response = UserAgentBase.open(self, request, data)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_opener.py", line 188, in open
req = meth(req)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 173, in http_request
self.rfp.read()
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 106, in read
line = f.readline()
UnboundLocalError: local variable 'f' referenced before assignment
the URL is a local machine with basic HTML and PHP code exactly like this
I tried simply running
formSubmit.open("http://172.16.142.154/index.html")
still shows same error. what should I do ?
Reference
https://cyberpersons.com/2016/12/04/automate-cross-site-scripting-xss-attack-using-beautiful-soup-mechanize/
python python-3.x url beautifulsoup
$endgroup$
add a comment |
$begingroup$
I am trying to make a python script that can find Cross-Site Script vulnerability on a web page.
First, it will fetch all the <input> tag from a <form> in a webpage.
Puts the input field's name in a list "inputFieldName".
At last, I have hardcoded the position of the payload.
import requests
import mechanize
from bs4 import BeautifulSoup
request = requests.get("http://172.16.142.154/index.html")
parseHTML = BeautifulSoup(request.text, 'html.parser')
formName = parseHTML.form['name']
print("Form name: " + formName)
inputs = parseHTML.form.find_all('input')
inputFieldNames =
for items in inputs:
if items.has_attr('name'):
inputFieldNames.append(items['name'])
print (inputFieldNames)
#
formSubmit = mechanize.Browser()
formSubmit.open("http://172.16.142.154/index.html")
formSubmit.select_form(formName)
payload = '<script>alert("vulnerable")</script>'
formSubmit.form[inputFieldNames[0]] = payload
formSubmit.form[inputFieldNames[1]] = "akshansh@shs.com"
formSubmit.submit()
print(formSubmit.response().read())
finalResult = formSubmit.response().read()
if finalResult.find('<script>') >= 0:
print("Application is vulnerable")
else:
print("You are in good hands")
I have downloaded 3 packages: requests, bs4 and mechanize.
bs4 is working fine but mechanize is showing this error:
UnboundLocalError: local variable 'f' referenced before assignment
Mechanize package is used for navigating through web forms.
https://pypi.org/project/mechanize/
till print(inputFieldNames)
its working fine
full traceback:
Form name: HeheForm
['name', 'email']
Traceback (most recent call last):
File "xss.py", line 20, in <module>
formSubmit.open("http://172.16.142.154/index.html")
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 253, in open
return self._mech_open(url_or_request, data, timeout=timeout)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 283, in _mech_open
response = UserAgentBase.open(self, request, data)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_opener.py", line 188, in open
req = meth(req)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 173, in http_request
self.rfp.read()
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 106, in read
line = f.readline()
UnboundLocalError: local variable 'f' referenced before assignment
the URL is a local machine with basic HTML and PHP code exactly like this
I tried simply running
formSubmit.open("http://172.16.142.154/index.html")
still shows same error. what should I do ?
Reference
https://cyberpersons.com/2016/12/04/automate-cross-site-scripting-xss-attack-using-beautiful-soup-mechanize/
python python-3.x url beautifulsoup
$endgroup$
I am trying to make a python script that can find Cross-Site Script vulnerability on a web page.
First, it will fetch all the <input> tag from a <form> in a webpage.
Puts the input field's name in a list "inputFieldName".
At last, I have hardcoded the position of the payload.
import requests
import mechanize
from bs4 import BeautifulSoup
request = requests.get("http://172.16.142.154/index.html")
parseHTML = BeautifulSoup(request.text, 'html.parser')
formName = parseHTML.form['name']
print("Form name: " + formName)
inputs = parseHTML.form.find_all('input')
inputFieldNames =
for items in inputs:
if items.has_attr('name'):
inputFieldNames.append(items['name'])
print (inputFieldNames)
#
formSubmit = mechanize.Browser()
formSubmit.open("http://172.16.142.154/index.html")
formSubmit.select_form(formName)
payload = '<script>alert("vulnerable")</script>'
formSubmit.form[inputFieldNames[0]] = payload
formSubmit.form[inputFieldNames[1]] = "akshansh@shs.com"
formSubmit.submit()
print(formSubmit.response().read())
finalResult = formSubmit.response().read()
if finalResult.find('<script>') >= 0:
print("Application is vulnerable")
else:
print("You are in good hands")
I have downloaded 3 packages: requests, bs4 and mechanize.
bs4 is working fine but mechanize is showing this error:
UnboundLocalError: local variable 'f' referenced before assignment
Mechanize package is used for navigating through web forms.
https://pypi.org/project/mechanize/
till print(inputFieldNames)
its working fine
full traceback:
Form name: HeheForm
['name', 'email']
Traceback (most recent call last):
File "xss.py", line 20, in <module>
formSubmit.open("http://172.16.142.154/index.html")
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 253, in open
return self._mech_open(url_or_request, data, timeout=timeout)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 283, in _mech_open
response = UserAgentBase.open(self, request, data)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_opener.py", line 188, in open
req = meth(req)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 173, in http_request
self.rfp.read()
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 106, in read
line = f.readline()
UnboundLocalError: local variable 'f' referenced before assignment
the URL is a local machine with basic HTML and PHP code exactly like this
I tried simply running
formSubmit.open("http://172.16.142.154/index.html")
still shows same error. what should I do ?
Reference
https://cyberpersons.com/2016/12/04/automate-cross-site-scripting-xss-attack-using-beautiful-soup-mechanize/
Form name: HeheForm
['name', 'email']
Traceback (most recent call last):
File "xss.py", line 20, in <module>
formSubmit.open("http://172.16.142.154/index.html")
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 253, in open
return self._mech_open(url_or_request, data, timeout=timeout)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 283, in _mech_open
response = UserAgentBase.open(self, request, data)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_opener.py", line 188, in open
req = meth(req)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 173, in http_request
self.rfp.read()
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 106, in read
line = f.readline()
UnboundLocalError: local variable 'f' referenced before assignment
Form name: HeheForm
['name', 'email']
Traceback (most recent call last):
File "xss.py", line 20, in <module>
formSubmit.open("http://172.16.142.154/index.html")
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 253, in open
return self._mech_open(url_or_request, data, timeout=timeout)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_mechanize.py", line 283, in _mech_open
response = UserAgentBase.open(self, request, data)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_opener.py", line 188, in open
req = meth(req)
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 173, in http_request
self.rfp.read()
File "/Users/akshansh.s/PycharmProjects/test/venv/lib/python3.7/site-packages/mechanize/_http.py", line 106, in read
line = f.readline()
UnboundLocalError: local variable 'f' referenced before assignment
python python-3.x url beautifulsoup
python python-3.x url beautifulsoup
asked 9 mins ago
Akshansh ShrivastavaAkshansh Shrivastava
435
435
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f212957%2fpython-script-for-finding-xss-vulnerabilities-with-mechanize%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f212957%2fpython-script-for-finding-xss-vulnerabilities-with-mechanize%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3jAY8eS4JuIK9WD