Reverse for loop C++ warning message [on hold]
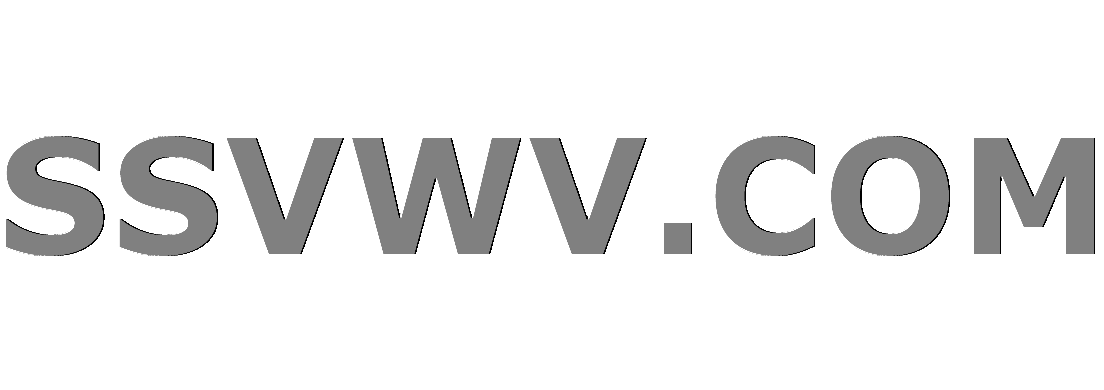
Multi tool use
up vote
-1
down vote
favorite
I would like to print a vector backwards, so I've created a function called reverse. The output is correct but I get this warning message:
Implicit conversion loses integer precision: 'std::__1::vector<int, std::__1::allocator<int> >::size_type' (aka 'unsigned long') to 'unsigned int'
I get this message from the for loop inside the reverseVector function.
Why?
This is my code:
#include <iostream>
#include <vector>
using namespace std;
// functions prototype:
void fillVector(vector<int>&);
void reverseVector(const vector<int>&);
int main(){
vector<int> myVector;
fillVector(myVector);
printVector(myVector);
reverseVector(myVector);
return 0;
}
//function definitions
void fillVector(vector<int>& newMyVector){
cout << "Type in a list of numbers (-1 to stop): ";
int input;
cin >> input;
while (input != -1) {
newMyVector.push_back(input);
cin >> input;
}
cout << endl;
}
void reverseVector(const vector<int>& newMyVector){
for (unsigned int k = newMyVector.size(); k > 0 ; k = k - 1) {
cout << newMyVector[k - 1] << " ";
}
cout << endl;
}
c++
New contributor
Fredo is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by Sᴀᴍ Onᴇᴌᴀ, Malachi♦ yesterday
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Sᴀᴍ Onᴇᴌᴀ, Malachi
If this question can be reworded to fit the rules in the help center, please edit the question.
|
show 2 more comments
up vote
-1
down vote
favorite
I would like to print a vector backwards, so I've created a function called reverse. The output is correct but I get this warning message:
Implicit conversion loses integer precision: 'std::__1::vector<int, std::__1::allocator<int> >::size_type' (aka 'unsigned long') to 'unsigned int'
I get this message from the for loop inside the reverseVector function.
Why?
This is my code:
#include <iostream>
#include <vector>
using namespace std;
// functions prototype:
void fillVector(vector<int>&);
void reverseVector(const vector<int>&);
int main(){
vector<int> myVector;
fillVector(myVector);
printVector(myVector);
reverseVector(myVector);
return 0;
}
//function definitions
void fillVector(vector<int>& newMyVector){
cout << "Type in a list of numbers (-1 to stop): ";
int input;
cin >> input;
while (input != -1) {
newMyVector.push_back(input);
cin >> input;
}
cout << endl;
}
void reverseVector(const vector<int>& newMyVector){
for (unsigned int k = newMyVector.size(); k > 0 ; k = k - 1) {
cout << newMyVector[k - 1] << " ";
}
cout << endl;
}
c++
New contributor
Fredo is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by Sᴀᴍ Onᴇᴌᴀ, Malachi♦ yesterday
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Sᴀᴍ Onᴇᴌᴀ, Malachi
If this question can be reworded to fit the rules in the help center, please edit the question.
1
Why not just usestd::reverse
?
– Edward
yesterday
@Edward did not know about that function! :) Anyways I'm doing those "silly" functions to practise.
– Fredo
yesterday
Thesize()
function ofstd::vector<int>
returns astd::vector<int>::size_type
so the type ofk
should be that as well (or simply use theauto
keyword for it).
– Null
yesterday
@Null I'm confused, isn't the type of both int?
– Fredo
yesterday
No, you declaredk
to be anunsigned int
. You may be confusing thevalue_type
ofstd::vector<int>
(which is indeed anint
) with the return type ofstd::vector<int>::size()
.
– Null
yesterday
|
show 2 more comments
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I would like to print a vector backwards, so I've created a function called reverse. The output is correct but I get this warning message:
Implicit conversion loses integer precision: 'std::__1::vector<int, std::__1::allocator<int> >::size_type' (aka 'unsigned long') to 'unsigned int'
I get this message from the for loop inside the reverseVector function.
Why?
This is my code:
#include <iostream>
#include <vector>
using namespace std;
// functions prototype:
void fillVector(vector<int>&);
void reverseVector(const vector<int>&);
int main(){
vector<int> myVector;
fillVector(myVector);
printVector(myVector);
reverseVector(myVector);
return 0;
}
//function definitions
void fillVector(vector<int>& newMyVector){
cout << "Type in a list of numbers (-1 to stop): ";
int input;
cin >> input;
while (input != -1) {
newMyVector.push_back(input);
cin >> input;
}
cout << endl;
}
void reverseVector(const vector<int>& newMyVector){
for (unsigned int k = newMyVector.size(); k > 0 ; k = k - 1) {
cout << newMyVector[k - 1] << " ";
}
cout << endl;
}
c++
New contributor
Fredo is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I would like to print a vector backwards, so I've created a function called reverse. The output is correct but I get this warning message:
Implicit conversion loses integer precision: 'std::__1::vector<int, std::__1::allocator<int> >::size_type' (aka 'unsigned long') to 'unsigned int'
I get this message from the for loop inside the reverseVector function.
Why?
This is my code:
#include <iostream>
#include <vector>
using namespace std;
// functions prototype:
void fillVector(vector<int>&);
void reverseVector(const vector<int>&);
int main(){
vector<int> myVector;
fillVector(myVector);
printVector(myVector);
reverseVector(myVector);
return 0;
}
//function definitions
void fillVector(vector<int>& newMyVector){
cout << "Type in a list of numbers (-1 to stop): ";
int input;
cin >> input;
while (input != -1) {
newMyVector.push_back(input);
cin >> input;
}
cout << endl;
}
void reverseVector(const vector<int>& newMyVector){
for (unsigned int k = newMyVector.size(); k > 0 ; k = k - 1) {
cout << newMyVector[k - 1] << " ";
}
cout << endl;
}
c++
c++
New contributor
Fredo is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Fredo is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited yesterday
Deduplicator
10.8k1849
10.8k1849
New contributor
Fredo is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked yesterday


Fredo
12
12
New contributor
Fredo is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Fredo is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Fredo is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by Sᴀᴍ Onᴇᴌᴀ, Malachi♦ yesterday
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Sᴀᴍ Onᴇᴌᴀ, Malachi
If this question can be reworded to fit the rules in the help center, please edit the question.
put on hold as off-topic by Sᴀᴍ Onᴇᴌᴀ, Malachi♦ yesterday
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "Code not implemented or not working as intended: Code Review is a community where programmers peer-review your working code to address issues such as security, maintainability, performance, and scalability. We require that the code be working correctly, to the best of the author's knowledge, before proceeding with a review." – Sᴀᴍ Onᴇᴌᴀ, Malachi
If this question can be reworded to fit the rules in the help center, please edit the question.
1
Why not just usestd::reverse
?
– Edward
yesterday
@Edward did not know about that function! :) Anyways I'm doing those "silly" functions to practise.
– Fredo
yesterday
Thesize()
function ofstd::vector<int>
returns astd::vector<int>::size_type
so the type ofk
should be that as well (or simply use theauto
keyword for it).
– Null
yesterday
@Null I'm confused, isn't the type of both int?
– Fredo
yesterday
No, you declaredk
to be anunsigned int
. You may be confusing thevalue_type
ofstd::vector<int>
(which is indeed anint
) with the return type ofstd::vector<int>::size()
.
– Null
yesterday
|
show 2 more comments
1
Why not just usestd::reverse
?
– Edward
yesterday
@Edward did not know about that function! :) Anyways I'm doing those "silly" functions to practise.
– Fredo
yesterday
Thesize()
function ofstd::vector<int>
returns astd::vector<int>::size_type
so the type ofk
should be that as well (or simply use theauto
keyword for it).
– Null
yesterday
@Null I'm confused, isn't the type of both int?
– Fredo
yesterday
No, you declaredk
to be anunsigned int
. You may be confusing thevalue_type
ofstd::vector<int>
(which is indeed anint
) with the return type ofstd::vector<int>::size()
.
– Null
yesterday
1
1
Why not just use
std::reverse
?– Edward
yesterday
Why not just use
std::reverse
?– Edward
yesterday
@Edward did not know about that function! :) Anyways I'm doing those "silly" functions to practise.
– Fredo
yesterday
@Edward did not know about that function! :) Anyways I'm doing those "silly" functions to practise.
– Fredo
yesterday
The
size()
function of std::vector<int>
returns a std::vector<int>::size_type
so the type of k
should be that as well (or simply use the auto
keyword for it).– Null
yesterday
The
size()
function of std::vector<int>
returns a std::vector<int>::size_type
so the type of k
should be that as well (or simply use the auto
keyword for it).– Null
yesterday
@Null I'm confused, isn't the type of both int?
– Fredo
yesterday
@Null I'm confused, isn't the type of both int?
– Fredo
yesterday
No, you declared
k
to be an unsigned int
. You may be confusing the value_type
of std::vector<int>
(which is indeed an int
) with the return type of std::vector<int>::size()
.– Null
yesterday
No, you declared
k
to be an unsigned int
. You may be confusing the value_type
of std::vector<int>
(which is indeed an int
) with the return type of std::vector<int>::size()
.– Null
yesterday
|
show 2 more comments
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
Avoid using namespace std
It's harmful to include all identifiers from namespaces that are not designed for it. Use just what you need, where you need it - in most cases, it's easier to fully qualify the names you use.
Iterators can be easier than indexing
Instead of
// bug fixed (was: unsigned int k)
for (auto k = newMyVector.size(); k > 0; k = k - 1) {
std::cout << newMyVector[k - 1] << " ";
}
look at the iterator equivalent:
for (auto it = newMyVector.rbegin(); it != newMyVector.rend(); ++it) {
std::cout << *it << " ";
}
There's almost no arithmetic to get wrong here. It would be clearer still with a shorter name for the vector.
Consider allowing other streams
At the moment, we always use the standard input and output streams. What would you need to do, to allow your program to read and write from/to other streams (such as file streams)?
1
Better, I think, would bestd::copy(vec.rbegin(), vec.rend(), std::ostream_iterator(std::cout, " "));
because it's more direct and sometimes confers a performance improvement.
– Edward
yesterday
Agreed - and even less arithmetic!
– Toby Speight
yesterday
I can also define: unsigned int size = newMyVector.size(), before the for lop, so the loop became: for (size; k > 0 ; k = k - 1){statements}. Right?
– Fredo
yesterday
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Avoid using namespace std
It's harmful to include all identifiers from namespaces that are not designed for it. Use just what you need, where you need it - in most cases, it's easier to fully qualify the names you use.
Iterators can be easier than indexing
Instead of
// bug fixed (was: unsigned int k)
for (auto k = newMyVector.size(); k > 0; k = k - 1) {
std::cout << newMyVector[k - 1] << " ";
}
look at the iterator equivalent:
for (auto it = newMyVector.rbegin(); it != newMyVector.rend(); ++it) {
std::cout << *it << " ";
}
There's almost no arithmetic to get wrong here. It would be clearer still with a shorter name for the vector.
Consider allowing other streams
At the moment, we always use the standard input and output streams. What would you need to do, to allow your program to read and write from/to other streams (such as file streams)?
1
Better, I think, would bestd::copy(vec.rbegin(), vec.rend(), std::ostream_iterator(std::cout, " "));
because it's more direct and sometimes confers a performance improvement.
– Edward
yesterday
Agreed - and even less arithmetic!
– Toby Speight
yesterday
I can also define: unsigned int size = newMyVector.size(), before the for lop, so the loop became: for (size; k > 0 ; k = k - 1){statements}. Right?
– Fredo
yesterday
add a comment |
up vote
1
down vote
accepted
Avoid using namespace std
It's harmful to include all identifiers from namespaces that are not designed for it. Use just what you need, where you need it - in most cases, it's easier to fully qualify the names you use.
Iterators can be easier than indexing
Instead of
// bug fixed (was: unsigned int k)
for (auto k = newMyVector.size(); k > 0; k = k - 1) {
std::cout << newMyVector[k - 1] << " ";
}
look at the iterator equivalent:
for (auto it = newMyVector.rbegin(); it != newMyVector.rend(); ++it) {
std::cout << *it << " ";
}
There's almost no arithmetic to get wrong here. It would be clearer still with a shorter name for the vector.
Consider allowing other streams
At the moment, we always use the standard input and output streams. What would you need to do, to allow your program to read and write from/to other streams (such as file streams)?
1
Better, I think, would bestd::copy(vec.rbegin(), vec.rend(), std::ostream_iterator(std::cout, " "));
because it's more direct and sometimes confers a performance improvement.
– Edward
yesterday
Agreed - and even less arithmetic!
– Toby Speight
yesterday
I can also define: unsigned int size = newMyVector.size(), before the for lop, so the loop became: for (size; k > 0 ; k = k - 1){statements}. Right?
– Fredo
yesterday
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Avoid using namespace std
It's harmful to include all identifiers from namespaces that are not designed for it. Use just what you need, where you need it - in most cases, it's easier to fully qualify the names you use.
Iterators can be easier than indexing
Instead of
// bug fixed (was: unsigned int k)
for (auto k = newMyVector.size(); k > 0; k = k - 1) {
std::cout << newMyVector[k - 1] << " ";
}
look at the iterator equivalent:
for (auto it = newMyVector.rbegin(); it != newMyVector.rend(); ++it) {
std::cout << *it << " ";
}
There's almost no arithmetic to get wrong here. It would be clearer still with a shorter name for the vector.
Consider allowing other streams
At the moment, we always use the standard input and output streams. What would you need to do, to allow your program to read and write from/to other streams (such as file streams)?
Avoid using namespace std
It's harmful to include all identifiers from namespaces that are not designed for it. Use just what you need, where you need it - in most cases, it's easier to fully qualify the names you use.
Iterators can be easier than indexing
Instead of
// bug fixed (was: unsigned int k)
for (auto k = newMyVector.size(); k > 0; k = k - 1) {
std::cout << newMyVector[k - 1] << " ";
}
look at the iterator equivalent:
for (auto it = newMyVector.rbegin(); it != newMyVector.rend(); ++it) {
std::cout << *it << " ";
}
There's almost no arithmetic to get wrong here. It would be clearer still with a shorter name for the vector.
Consider allowing other streams
At the moment, we always use the standard input and output streams. What would you need to do, to allow your program to read and write from/to other streams (such as file streams)?
answered yesterday
Toby Speight
21.8k536107
21.8k536107
1
Better, I think, would bestd::copy(vec.rbegin(), vec.rend(), std::ostream_iterator(std::cout, " "));
because it's more direct and sometimes confers a performance improvement.
– Edward
yesterday
Agreed - and even less arithmetic!
– Toby Speight
yesterday
I can also define: unsigned int size = newMyVector.size(), before the for lop, so the loop became: for (size; k > 0 ; k = k - 1){statements}. Right?
– Fredo
yesterday
add a comment |
1
Better, I think, would bestd::copy(vec.rbegin(), vec.rend(), std::ostream_iterator(std::cout, " "));
because it's more direct and sometimes confers a performance improvement.
– Edward
yesterday
Agreed - and even less arithmetic!
– Toby Speight
yesterday
I can also define: unsigned int size = newMyVector.size(), before the for lop, so the loop became: for (size; k > 0 ; k = k - 1){statements}. Right?
– Fredo
yesterday
1
1
Better, I think, would be
std::copy(vec.rbegin(), vec.rend(), std::ostream_iterator(std::cout, " "));
because it's more direct and sometimes confers a performance improvement.– Edward
yesterday
Better, I think, would be
std::copy(vec.rbegin(), vec.rend(), std::ostream_iterator(std::cout, " "));
because it's more direct and sometimes confers a performance improvement.– Edward
yesterday
Agreed - and even less arithmetic!
– Toby Speight
yesterday
Agreed - and even less arithmetic!
– Toby Speight
yesterday
I can also define: unsigned int size = newMyVector.size(), before the for lop, so the loop became: for (size; k > 0 ; k = k - 1){statements}. Right?
– Fredo
yesterday
I can also define: unsigned int size = newMyVector.size(), before the for lop, so the loop became: for (size; k > 0 ; k = k - 1){statements}. Right?
– Fredo
yesterday
add a comment |
TKXcbhdp 52sgfWaubzFj0ESqJl
1
Why not just use
std::reverse
?– Edward
yesterday
@Edward did not know about that function! :) Anyways I'm doing those "silly" functions to practise.
– Fredo
yesterday
The
size()
function ofstd::vector<int>
returns astd::vector<int>::size_type
so the type ofk
should be that as well (or simply use theauto
keyword for it).– Null
yesterday
@Null I'm confused, isn't the type of both int?
– Fredo
yesterday
No, you declared
k
to be anunsigned int
. You may be confusing thevalue_type
ofstd::vector<int>
(which is indeed anint
) with the return type ofstd::vector<int>::size()
.– Null
yesterday