Program to check if heap order is correct or not
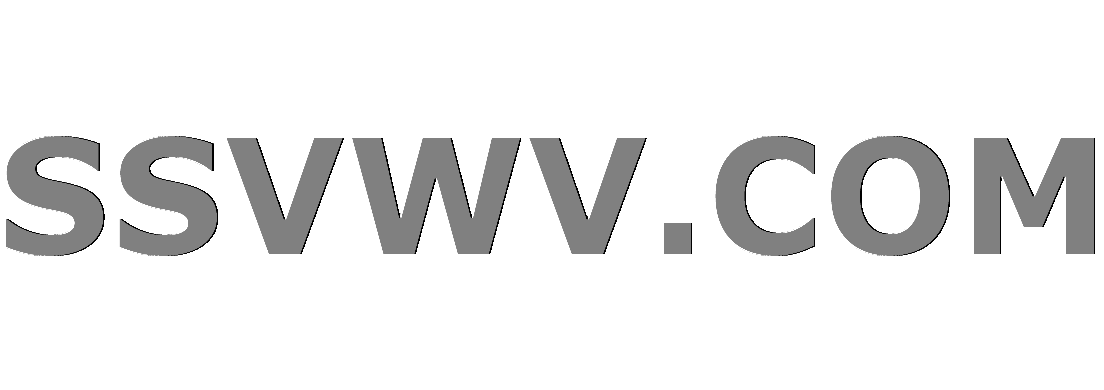
Multi tool use
There is a Java program to check if a Heap (Min) is in the correct order. Heap Order means the parent is less than both left child and right child.
package testers;
import heap.Heap;
import java.util.List;
public class HeapTester
{
public static void main(String args)
{
Heap<Integer> heap = new Heap <>();
heap.add(50);
heap.add(25);
heap.add(26);
heap.add(15);
heap.add(10);
heap.add(16);
heap.add(3);
heap.add(7);
heap.add(10);
heap.add(12);
heap.add(2);
System.out.println(heap);
System.out.println(hasHeapOrder(heap.copy()));
}
private static <T extends Comparable<T>> boolean hasHeapOrder(List<T> list)
{
if(list.size() < 2) return true;
boolean hasOrder = true;
int parent = 0;
int leftChild = 2 * parent + 1;
int rightChild = 2 * parent + 2;
while(leftChild < list.size())
{
if(list.get(parent).compareTo(list.get(leftChild)) > 0)
{
hasOrder = false;
break;
}
if(rightChild < list.size())
{
if(list.get(parent).compareTo(list.get(rightChild)) > 0)
{
hasOrder = false;
break;
}
}
parent++;
leftChild = 2 * parent + 1;
rightChild = 2 * parent + 2;
}
return hasOrder;
}
}
java generics heap
add a comment |
There is a Java program to check if a Heap (Min) is in the correct order. Heap Order means the parent is less than both left child and right child.
package testers;
import heap.Heap;
import java.util.List;
public class HeapTester
{
public static void main(String args)
{
Heap<Integer> heap = new Heap <>();
heap.add(50);
heap.add(25);
heap.add(26);
heap.add(15);
heap.add(10);
heap.add(16);
heap.add(3);
heap.add(7);
heap.add(10);
heap.add(12);
heap.add(2);
System.out.println(heap);
System.out.println(hasHeapOrder(heap.copy()));
}
private static <T extends Comparable<T>> boolean hasHeapOrder(List<T> list)
{
if(list.size() < 2) return true;
boolean hasOrder = true;
int parent = 0;
int leftChild = 2 * parent + 1;
int rightChild = 2 * parent + 2;
while(leftChild < list.size())
{
if(list.get(parent).compareTo(list.get(leftChild)) > 0)
{
hasOrder = false;
break;
}
if(rightChild < list.size())
{
if(list.get(parent).compareTo(list.get(rightChild)) > 0)
{
hasOrder = false;
break;
}
}
parent++;
leftChild = 2 * parent + 1;
rightChild = 2 * parent + 2;
}
return hasOrder;
}
}
java generics heap
add a comment |
There is a Java program to check if a Heap (Min) is in the correct order. Heap Order means the parent is less than both left child and right child.
package testers;
import heap.Heap;
import java.util.List;
public class HeapTester
{
public static void main(String args)
{
Heap<Integer> heap = new Heap <>();
heap.add(50);
heap.add(25);
heap.add(26);
heap.add(15);
heap.add(10);
heap.add(16);
heap.add(3);
heap.add(7);
heap.add(10);
heap.add(12);
heap.add(2);
System.out.println(heap);
System.out.println(hasHeapOrder(heap.copy()));
}
private static <T extends Comparable<T>> boolean hasHeapOrder(List<T> list)
{
if(list.size() < 2) return true;
boolean hasOrder = true;
int parent = 0;
int leftChild = 2 * parent + 1;
int rightChild = 2 * parent + 2;
while(leftChild < list.size())
{
if(list.get(parent).compareTo(list.get(leftChild)) > 0)
{
hasOrder = false;
break;
}
if(rightChild < list.size())
{
if(list.get(parent).compareTo(list.get(rightChild)) > 0)
{
hasOrder = false;
break;
}
}
parent++;
leftChild = 2 * parent + 1;
rightChild = 2 * parent + 2;
}
return hasOrder;
}
}
java generics heap
There is a Java program to check if a Heap (Min) is in the correct order. Heap Order means the parent is less than both left child and right child.
package testers;
import heap.Heap;
import java.util.List;
public class HeapTester
{
public static void main(String args)
{
Heap<Integer> heap = new Heap <>();
heap.add(50);
heap.add(25);
heap.add(26);
heap.add(15);
heap.add(10);
heap.add(16);
heap.add(3);
heap.add(7);
heap.add(10);
heap.add(12);
heap.add(2);
System.out.println(heap);
System.out.println(hasHeapOrder(heap.copy()));
}
private static <T extends Comparable<T>> boolean hasHeapOrder(List<T> list)
{
if(list.size() < 2) return true;
boolean hasOrder = true;
int parent = 0;
int leftChild = 2 * parent + 1;
int rightChild = 2 * parent + 2;
while(leftChild < list.size())
{
if(list.get(parent).compareTo(list.get(leftChild)) > 0)
{
hasOrder = false;
break;
}
if(rightChild < list.size())
{
if(list.get(parent).compareTo(list.get(rightChild)) > 0)
{
hasOrder = false;
break;
}
}
parent++;
leftChild = 2 * parent + 1;
rightChild = 2 * parent + 2;
}
return hasOrder;
}
}
java generics heap
java generics heap
edited Dec 16 at 18:19
mdfst13
17.1k52155
17.1k52155
asked Dec 16 at 18:18


Hamidur Rahman
536
536
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Avoid flag variables if you don't need them
The hasOrder
flag variable is unnecessary.
When you set this variable to false
,
you could return false
instead.
If the end of the loop is reached, you can return true
.
Avoid unnecessary special handling
The special treatment for the case list.size() < 2
is unnecessary.
The rest of the implementation handles that case naturally.
Don't repeat yourself
The computation logic of the left and right child indexes is duplicated twice.
You could eliminate that by changing the loop to while (true)
, and making this computation the first step of the loop instead of last:
while (true) {
int leftChild = 2 * parent + 1;
int rightChild = 2 * parent + 2;
if (leftChild >= list.size()) {
break;
}
// ...
Test using a unit test framework
Testing by printing stuff is not very useful.
You have to read the output to verify it's correct,
which takes mental effort, and error-prone.
It's better to use a proper unit testing framework,
where test cases will give you simple yes-no answers per test case;
no need to re-interpret passing results.
Test all corner cases
Only one case is "tested".
You need more tests to verify that hasHeapOrder
correctly returns true
or false
depending on the input.
I suppose you have an implementation of Heap
such that heap.copy()
returns a correctly heap-ordered list, and so hasHeapOrder
returns true
.
At the minimum,
you should verify that hasHeapOrder
returns false
for lists like 4, 1, 2 and 4, 5, 2.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f209778%2fprogram-to-check-if-heap-order-is-correct-or-not%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Avoid flag variables if you don't need them
The hasOrder
flag variable is unnecessary.
When you set this variable to false
,
you could return false
instead.
If the end of the loop is reached, you can return true
.
Avoid unnecessary special handling
The special treatment for the case list.size() < 2
is unnecessary.
The rest of the implementation handles that case naturally.
Don't repeat yourself
The computation logic of the left and right child indexes is duplicated twice.
You could eliminate that by changing the loop to while (true)
, and making this computation the first step of the loop instead of last:
while (true) {
int leftChild = 2 * parent + 1;
int rightChild = 2 * parent + 2;
if (leftChild >= list.size()) {
break;
}
// ...
Test using a unit test framework
Testing by printing stuff is not very useful.
You have to read the output to verify it's correct,
which takes mental effort, and error-prone.
It's better to use a proper unit testing framework,
where test cases will give you simple yes-no answers per test case;
no need to re-interpret passing results.
Test all corner cases
Only one case is "tested".
You need more tests to verify that hasHeapOrder
correctly returns true
or false
depending on the input.
I suppose you have an implementation of Heap
such that heap.copy()
returns a correctly heap-ordered list, and so hasHeapOrder
returns true
.
At the minimum,
you should verify that hasHeapOrder
returns false
for lists like 4, 1, 2 and 4, 5, 2.
add a comment |
Avoid flag variables if you don't need them
The hasOrder
flag variable is unnecessary.
When you set this variable to false
,
you could return false
instead.
If the end of the loop is reached, you can return true
.
Avoid unnecessary special handling
The special treatment for the case list.size() < 2
is unnecessary.
The rest of the implementation handles that case naturally.
Don't repeat yourself
The computation logic of the left and right child indexes is duplicated twice.
You could eliminate that by changing the loop to while (true)
, and making this computation the first step of the loop instead of last:
while (true) {
int leftChild = 2 * parent + 1;
int rightChild = 2 * parent + 2;
if (leftChild >= list.size()) {
break;
}
// ...
Test using a unit test framework
Testing by printing stuff is not very useful.
You have to read the output to verify it's correct,
which takes mental effort, and error-prone.
It's better to use a proper unit testing framework,
where test cases will give you simple yes-no answers per test case;
no need to re-interpret passing results.
Test all corner cases
Only one case is "tested".
You need more tests to verify that hasHeapOrder
correctly returns true
or false
depending on the input.
I suppose you have an implementation of Heap
such that heap.copy()
returns a correctly heap-ordered list, and so hasHeapOrder
returns true
.
At the minimum,
you should verify that hasHeapOrder
returns false
for lists like 4, 1, 2 and 4, 5, 2.
add a comment |
Avoid flag variables if you don't need them
The hasOrder
flag variable is unnecessary.
When you set this variable to false
,
you could return false
instead.
If the end of the loop is reached, you can return true
.
Avoid unnecessary special handling
The special treatment for the case list.size() < 2
is unnecessary.
The rest of the implementation handles that case naturally.
Don't repeat yourself
The computation logic of the left and right child indexes is duplicated twice.
You could eliminate that by changing the loop to while (true)
, and making this computation the first step of the loop instead of last:
while (true) {
int leftChild = 2 * parent + 1;
int rightChild = 2 * parent + 2;
if (leftChild >= list.size()) {
break;
}
// ...
Test using a unit test framework
Testing by printing stuff is not very useful.
You have to read the output to verify it's correct,
which takes mental effort, and error-prone.
It's better to use a proper unit testing framework,
where test cases will give you simple yes-no answers per test case;
no need to re-interpret passing results.
Test all corner cases
Only one case is "tested".
You need more tests to verify that hasHeapOrder
correctly returns true
or false
depending on the input.
I suppose you have an implementation of Heap
such that heap.copy()
returns a correctly heap-ordered list, and so hasHeapOrder
returns true
.
At the minimum,
you should verify that hasHeapOrder
returns false
for lists like 4, 1, 2 and 4, 5, 2.
Avoid flag variables if you don't need them
The hasOrder
flag variable is unnecessary.
When you set this variable to false
,
you could return false
instead.
If the end of the loop is reached, you can return true
.
Avoid unnecessary special handling
The special treatment for the case list.size() < 2
is unnecessary.
The rest of the implementation handles that case naturally.
Don't repeat yourself
The computation logic of the left and right child indexes is duplicated twice.
You could eliminate that by changing the loop to while (true)
, and making this computation the first step of the loop instead of last:
while (true) {
int leftChild = 2 * parent + 1;
int rightChild = 2 * parent + 2;
if (leftChild >= list.size()) {
break;
}
// ...
Test using a unit test framework
Testing by printing stuff is not very useful.
You have to read the output to verify it's correct,
which takes mental effort, and error-prone.
It's better to use a proper unit testing framework,
where test cases will give you simple yes-no answers per test case;
no need to re-interpret passing results.
Test all corner cases
Only one case is "tested".
You need more tests to verify that hasHeapOrder
correctly returns true
or false
depending on the input.
I suppose you have an implementation of Heap
such that heap.copy()
returns a correctly heap-ordered list, and so hasHeapOrder
returns true
.
At the minimum,
you should verify that hasHeapOrder
returns false
for lists like 4, 1, 2 and 4, 5, 2.
answered Dec 16 at 20:50


janos
97.1k12124350
97.1k12124350
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f209778%2fprogram-to-check-if-heap-order-is-correct-or-not%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zNzJYlNew96srSmLZPVrT,5 St5W7R6IXXwidC