Laravel function to add a supplier, and associated addresses and contacts
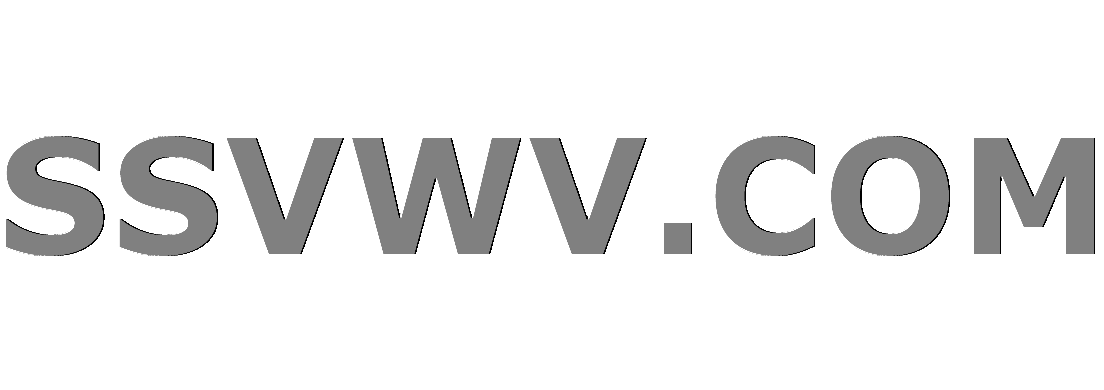
Multi tool use
up vote
0
down vote
favorite
I have a function addSupplier()
that adds a supplier. Each supplier has multiple addresses and each address has multiple contacts.
public function addSupplier ($request) {
// ADDING THE SUPPLIER BASIC INFO
$supplier_fillable_values = array_merge(
$request -> except('address_contact_inputs'),
['created_by' => $this->getAuthUserId()]
);
$new_supplier = Supplier::create($supplier_fillable_values);
// ADDING THE SUPPLIER ADDRESSES AND THE ADDRESS CONTACTS
foreach ($request -> address_contact_inputs as $address) {
// ADD ADDRESS
$new_supplier_address = $new_supplier -> addresses() -> create([
'address' => $address['address'],
'created_by' => $this -> getAuthUserId()
]);
// ADD ADDRESS CONTACTS
foreach ($address['contacts'] as $contact)
$new_supplier_address -> contacts() -> create([
'name' => $contact['name'],
'phone_number' => $contact['phone_number'],
'created_by' => $this -> getAuthUserId()
]);
}
return $request -> address_contact_inputs;
}
Everything works fine but question is: Is this the best practice or I should use events and create an event for:
- Storing the supplier basic info
- Storing the supplier addresses
- Storing contact for each supplier's address
php mvc laravel repository
add a comment |
up vote
0
down vote
favorite
I have a function addSupplier()
that adds a supplier. Each supplier has multiple addresses and each address has multiple contacts.
public function addSupplier ($request) {
// ADDING THE SUPPLIER BASIC INFO
$supplier_fillable_values = array_merge(
$request -> except('address_contact_inputs'),
['created_by' => $this->getAuthUserId()]
);
$new_supplier = Supplier::create($supplier_fillable_values);
// ADDING THE SUPPLIER ADDRESSES AND THE ADDRESS CONTACTS
foreach ($request -> address_contact_inputs as $address) {
// ADD ADDRESS
$new_supplier_address = $new_supplier -> addresses() -> create([
'address' => $address['address'],
'created_by' => $this -> getAuthUserId()
]);
// ADD ADDRESS CONTACTS
foreach ($address['contacts'] as $contact)
$new_supplier_address -> contacts() -> create([
'name' => $contact['name'],
'phone_number' => $contact['phone_number'],
'created_by' => $this -> getAuthUserId()
]);
}
return $request -> address_contact_inputs;
}
Everything works fine but question is: Is this the best practice or I should use events and create an event for:
- Storing the supplier basic info
- Storing the supplier addresses
- Storing contact for each supplier's address
php mvc laravel repository
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a function addSupplier()
that adds a supplier. Each supplier has multiple addresses and each address has multiple contacts.
public function addSupplier ($request) {
// ADDING THE SUPPLIER BASIC INFO
$supplier_fillable_values = array_merge(
$request -> except('address_contact_inputs'),
['created_by' => $this->getAuthUserId()]
);
$new_supplier = Supplier::create($supplier_fillable_values);
// ADDING THE SUPPLIER ADDRESSES AND THE ADDRESS CONTACTS
foreach ($request -> address_contact_inputs as $address) {
// ADD ADDRESS
$new_supplier_address = $new_supplier -> addresses() -> create([
'address' => $address['address'],
'created_by' => $this -> getAuthUserId()
]);
// ADD ADDRESS CONTACTS
foreach ($address['contacts'] as $contact)
$new_supplier_address -> contacts() -> create([
'name' => $contact['name'],
'phone_number' => $contact['phone_number'],
'created_by' => $this -> getAuthUserId()
]);
}
return $request -> address_contact_inputs;
}
Everything works fine but question is: Is this the best practice or I should use events and create an event for:
- Storing the supplier basic info
- Storing the supplier addresses
- Storing contact for each supplier's address
php mvc laravel repository
I have a function addSupplier()
that adds a supplier. Each supplier has multiple addresses and each address has multiple contacts.
public function addSupplier ($request) {
// ADDING THE SUPPLIER BASIC INFO
$supplier_fillable_values = array_merge(
$request -> except('address_contact_inputs'),
['created_by' => $this->getAuthUserId()]
);
$new_supplier = Supplier::create($supplier_fillable_values);
// ADDING THE SUPPLIER ADDRESSES AND THE ADDRESS CONTACTS
foreach ($request -> address_contact_inputs as $address) {
// ADD ADDRESS
$new_supplier_address = $new_supplier -> addresses() -> create([
'address' => $address['address'],
'created_by' => $this -> getAuthUserId()
]);
// ADD ADDRESS CONTACTS
foreach ($address['contacts'] as $contact)
$new_supplier_address -> contacts() -> create([
'name' => $contact['name'],
'phone_number' => $contact['phone_number'],
'created_by' => $this -> getAuthUserId()
]);
}
return $request -> address_contact_inputs;
}
Everything works fine but question is: Is this the best practice or I should use events and create an event for:
- Storing the supplier basic info
- Storing the supplier addresses
- Storing contact for each supplier's address
php mvc laravel repository
php mvc laravel repository
edited Oct 30 at 16:17


200_success
127k15148410
127k15148410
asked Oct 30 at 15:54
Ahmed essam
11
11
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You could use events for triggering these kinds of things, but there is a danger that your code starts to become harder to follow. Martin Fowler gives a good top-level talk on it here.
There are two issues jumping out at me:
Firstly, your method accepts a request object so there is no indication as to what the requirements of that method are. You could change the method to accept specific arguments such as $created_by, $address_details, etc. This would make it easier to re-use the code elsewhere if needed.
I would make the controller then responsible for preparing that set of data (e.g. handling the preparation of the address details array, getting the 'created_by' etc). And make your addSupplier method work with a set of arguments that could be run from anywhere in your app.
public function addSupplier ($primary_contact_details, $contact_addresses, $created_by = NULL) {
// ADDING THE SUPPLIER BASIC INFO
$supplier_fillable_values = array_merge(
$primary_contact_details,
['created_by' => $created_by]
);
$new_supplier = Supplier::create($supplier_fillable_values);
// ADDING THE SUPPLIER ADDRESSES AND THE ADDRESS CONTACTS
foreach ($contact_addresses as $address) {
// ADD ADDRESS
$new_supplier_address = $new_supplier -> addresses() -> create([
'address' => $address['address'],
'created_by' => $this -> getAuthUserId()
]);
// ADD ADDRESS CONTACTS
foreach ($address['contacts'] as $contact)
$new_supplier_address -> contacts() -> create([
'name' => $contact['name'],
'phone_number' => $contact['phone_number'],
'created_by' => $this -> getAuthUserId()
]);
}
return $request -> address_contact_inputs;
}
Secondly, it's good practise to avoid too many levels of indention, so you could refactor to use one level of indentation and splitting out the foreach loops to their own public/private methods on the same class.
New contributor
benjaminhull is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You could use events for triggering these kinds of things, but there is a danger that your code starts to become harder to follow. Martin Fowler gives a good top-level talk on it here.
There are two issues jumping out at me:
Firstly, your method accepts a request object so there is no indication as to what the requirements of that method are. You could change the method to accept specific arguments such as $created_by, $address_details, etc. This would make it easier to re-use the code elsewhere if needed.
I would make the controller then responsible for preparing that set of data (e.g. handling the preparation of the address details array, getting the 'created_by' etc). And make your addSupplier method work with a set of arguments that could be run from anywhere in your app.
public function addSupplier ($primary_contact_details, $contact_addresses, $created_by = NULL) {
// ADDING THE SUPPLIER BASIC INFO
$supplier_fillable_values = array_merge(
$primary_contact_details,
['created_by' => $created_by]
);
$new_supplier = Supplier::create($supplier_fillable_values);
// ADDING THE SUPPLIER ADDRESSES AND THE ADDRESS CONTACTS
foreach ($contact_addresses as $address) {
// ADD ADDRESS
$new_supplier_address = $new_supplier -> addresses() -> create([
'address' => $address['address'],
'created_by' => $this -> getAuthUserId()
]);
// ADD ADDRESS CONTACTS
foreach ($address['contacts'] as $contact)
$new_supplier_address -> contacts() -> create([
'name' => $contact['name'],
'phone_number' => $contact['phone_number'],
'created_by' => $this -> getAuthUserId()
]);
}
return $request -> address_contact_inputs;
}
Secondly, it's good practise to avoid too many levels of indention, so you could refactor to use one level of indentation and splitting out the foreach loops to their own public/private methods on the same class.
New contributor
benjaminhull is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
You could use events for triggering these kinds of things, but there is a danger that your code starts to become harder to follow. Martin Fowler gives a good top-level talk on it here.
There are two issues jumping out at me:
Firstly, your method accepts a request object so there is no indication as to what the requirements of that method are. You could change the method to accept specific arguments such as $created_by, $address_details, etc. This would make it easier to re-use the code elsewhere if needed.
I would make the controller then responsible for preparing that set of data (e.g. handling the preparation of the address details array, getting the 'created_by' etc). And make your addSupplier method work with a set of arguments that could be run from anywhere in your app.
public function addSupplier ($primary_contact_details, $contact_addresses, $created_by = NULL) {
// ADDING THE SUPPLIER BASIC INFO
$supplier_fillable_values = array_merge(
$primary_contact_details,
['created_by' => $created_by]
);
$new_supplier = Supplier::create($supplier_fillable_values);
// ADDING THE SUPPLIER ADDRESSES AND THE ADDRESS CONTACTS
foreach ($contact_addresses as $address) {
// ADD ADDRESS
$new_supplier_address = $new_supplier -> addresses() -> create([
'address' => $address['address'],
'created_by' => $this -> getAuthUserId()
]);
// ADD ADDRESS CONTACTS
foreach ($address['contacts'] as $contact)
$new_supplier_address -> contacts() -> create([
'name' => $contact['name'],
'phone_number' => $contact['phone_number'],
'created_by' => $this -> getAuthUserId()
]);
}
return $request -> address_contact_inputs;
}
Secondly, it's good practise to avoid too many levels of indention, so you could refactor to use one level of indentation and splitting out the foreach loops to their own public/private methods on the same class.
New contributor
benjaminhull is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
You could use events for triggering these kinds of things, but there is a danger that your code starts to become harder to follow. Martin Fowler gives a good top-level talk on it here.
There are two issues jumping out at me:
Firstly, your method accepts a request object so there is no indication as to what the requirements of that method are. You could change the method to accept specific arguments such as $created_by, $address_details, etc. This would make it easier to re-use the code elsewhere if needed.
I would make the controller then responsible for preparing that set of data (e.g. handling the preparation of the address details array, getting the 'created_by' etc). And make your addSupplier method work with a set of arguments that could be run from anywhere in your app.
public function addSupplier ($primary_contact_details, $contact_addresses, $created_by = NULL) {
// ADDING THE SUPPLIER BASIC INFO
$supplier_fillable_values = array_merge(
$primary_contact_details,
['created_by' => $created_by]
);
$new_supplier = Supplier::create($supplier_fillable_values);
// ADDING THE SUPPLIER ADDRESSES AND THE ADDRESS CONTACTS
foreach ($contact_addresses as $address) {
// ADD ADDRESS
$new_supplier_address = $new_supplier -> addresses() -> create([
'address' => $address['address'],
'created_by' => $this -> getAuthUserId()
]);
// ADD ADDRESS CONTACTS
foreach ($address['contacts'] as $contact)
$new_supplier_address -> contacts() -> create([
'name' => $contact['name'],
'phone_number' => $contact['phone_number'],
'created_by' => $this -> getAuthUserId()
]);
}
return $request -> address_contact_inputs;
}
Secondly, it's good practise to avoid too many levels of indention, so you could refactor to use one level of indentation and splitting out the foreach loops to their own public/private methods on the same class.
New contributor
benjaminhull is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
You could use events for triggering these kinds of things, but there is a danger that your code starts to become harder to follow. Martin Fowler gives a good top-level talk on it here.
There are two issues jumping out at me:
Firstly, your method accepts a request object so there is no indication as to what the requirements of that method are. You could change the method to accept specific arguments such as $created_by, $address_details, etc. This would make it easier to re-use the code elsewhere if needed.
I would make the controller then responsible for preparing that set of data (e.g. handling the preparation of the address details array, getting the 'created_by' etc). And make your addSupplier method work with a set of arguments that could be run from anywhere in your app.
public function addSupplier ($primary_contact_details, $contact_addresses, $created_by = NULL) {
// ADDING THE SUPPLIER BASIC INFO
$supplier_fillable_values = array_merge(
$primary_contact_details,
['created_by' => $created_by]
);
$new_supplier = Supplier::create($supplier_fillable_values);
// ADDING THE SUPPLIER ADDRESSES AND THE ADDRESS CONTACTS
foreach ($contact_addresses as $address) {
// ADD ADDRESS
$new_supplier_address = $new_supplier -> addresses() -> create([
'address' => $address['address'],
'created_by' => $this -> getAuthUserId()
]);
// ADD ADDRESS CONTACTS
foreach ($address['contacts'] as $contact)
$new_supplier_address -> contacts() -> create([
'name' => $contact['name'],
'phone_number' => $contact['phone_number'],
'created_by' => $this -> getAuthUserId()
]);
}
return $request -> address_contact_inputs;
}
Secondly, it's good practise to avoid too many levels of indention, so you could refactor to use one level of indentation and splitting out the foreach loops to their own public/private methods on the same class.
New contributor
benjaminhull is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 2 days ago
New contributor
benjaminhull is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 2 days ago
benjaminhull
61
61
New contributor
benjaminhull is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
benjaminhull is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
benjaminhull is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f206588%2flaravel-function-to-add-a-supplier-and-associated-addresses-and-contacts%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6z0Z7vWn,ShYDniTHt pzplg1Vft N1b,sVL8wZlassgl Ki,ILSX6,yqOhSv6P TGnjxjb61,yJMtZVIbkn7hD,jYwpNJts