Filtering queries based on the current user state
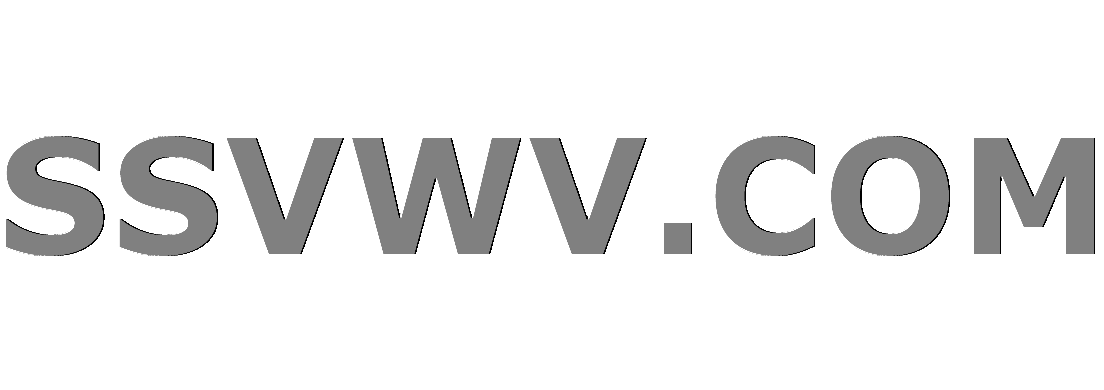
Multi tool use
up vote
4
down vote
favorite
I have a Symfony2 project. I have an Entity Asset
which can have relations with Category
. I store a categoryCount
-field within the Asset
to determine if an asset has one, to select those fast via a DQL-query depending on the current user state.
Now found myself writing something along the lines of this in my AssetRepository
:
/**
* @param string $orderBy
* @param int $mode
*
* @return DoctrineORMQuery
*/
public function getQueryForAll($orderBy = 'DESC', $mode = self::FILTER_WITHOUT_QUARANTINED)
{
$qb = $this->createQueryBuilder('asset');
$qb->orderBy('asset.updatedAt', $orderBy);
$filter = $this->getQuarantinedCriteria($mode);
if ($filter) {
$qb->addCriteria($filter);
}
return $query = $qb->getQuery();
}
/**
* @param int $mode
*
* @return Criteria|null
*/
protected function getQuarantinedCriteria($mode = self::FILTER_WITHOUT_QUARANTINED) {
$filter = null;
if ($mode === self::FILTER_WITHOUT_QUARANTINED) {
$filter = Criteria::create()
->andWhere(Criteria::expr()->gt('categoryCount', 0));
} else if ($mode === self::FILTER_ONLY_QURANTINED) {
$filter = Criteria::create()
->andWhere(Criteria::expr()->eq('categoryCount', 0));
}
return $filter;
}
This doesn't feel right to me. The $mode
flag triggers my code smell alarm, I also am worrying about passing it into each asset querying method.
Furthermore, since I will have to set the mode depending on the state of the current logged in user, I also am worried that I will produce code duplications by doing a lot of if-user-has-the-rights-checks in my actions or services:
/**
* @Route("/all_assets/{page}",
* name="get_paginated_assets",
* requirements={"page" = "d+"},
* defaults={"page" = "1"},
* options={"expose"=true}
* )
*/
public function getPaginatedAssetActions($page)
{
$repo = $this->getAssetRepository();
$isAllowedToViewRestrictedAssets = $this->getCurrentUser()->isAdmin();
if ($isAllowedToViewRestrictedAssets) {
$mode = EntityAssetRepository::FILTER_SHOW_ALL;
}
$query = $repo->getQueryForAll($mode);
$pagerfanta = new Pagerfanta(new DoctrineORMAdapter($query));
$data = $this->getCurrentPagedResults($pagerfanta, $page);
$view = $this->view();
$view->setFormat('json');
$view->setData($data);
return $view;
}
Is this the right way to implement the logic to enable filters in a Repository? Feedback on whether there is a more decoupled way would be highly appreciated.
php php5 doctrine
bumped to the homepage by Community♦ 3 hours ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
up vote
4
down vote
favorite
I have a Symfony2 project. I have an Entity Asset
which can have relations with Category
. I store a categoryCount
-field within the Asset
to determine if an asset has one, to select those fast via a DQL-query depending on the current user state.
Now found myself writing something along the lines of this in my AssetRepository
:
/**
* @param string $orderBy
* @param int $mode
*
* @return DoctrineORMQuery
*/
public function getQueryForAll($orderBy = 'DESC', $mode = self::FILTER_WITHOUT_QUARANTINED)
{
$qb = $this->createQueryBuilder('asset');
$qb->orderBy('asset.updatedAt', $orderBy);
$filter = $this->getQuarantinedCriteria($mode);
if ($filter) {
$qb->addCriteria($filter);
}
return $query = $qb->getQuery();
}
/**
* @param int $mode
*
* @return Criteria|null
*/
protected function getQuarantinedCriteria($mode = self::FILTER_WITHOUT_QUARANTINED) {
$filter = null;
if ($mode === self::FILTER_WITHOUT_QUARANTINED) {
$filter = Criteria::create()
->andWhere(Criteria::expr()->gt('categoryCount', 0));
} else if ($mode === self::FILTER_ONLY_QURANTINED) {
$filter = Criteria::create()
->andWhere(Criteria::expr()->eq('categoryCount', 0));
}
return $filter;
}
This doesn't feel right to me. The $mode
flag triggers my code smell alarm, I also am worrying about passing it into each asset querying method.
Furthermore, since I will have to set the mode depending on the state of the current logged in user, I also am worried that I will produce code duplications by doing a lot of if-user-has-the-rights-checks in my actions or services:
/**
* @Route("/all_assets/{page}",
* name="get_paginated_assets",
* requirements={"page" = "d+"},
* defaults={"page" = "1"},
* options={"expose"=true}
* )
*/
public function getPaginatedAssetActions($page)
{
$repo = $this->getAssetRepository();
$isAllowedToViewRestrictedAssets = $this->getCurrentUser()->isAdmin();
if ($isAllowedToViewRestrictedAssets) {
$mode = EntityAssetRepository::FILTER_SHOW_ALL;
}
$query = $repo->getQueryForAll($mode);
$pagerfanta = new Pagerfanta(new DoctrineORMAdapter($query));
$data = $this->getCurrentPagedResults($pagerfanta, $page);
$view = $this->view();
$view->setFormat('json');
$view->setData($data);
return $view;
}
Is this the right way to implement the logic to enable filters in a Repository? Feedback on whether there is a more decoupled way would be highly appreciated.
php php5 doctrine
bumped to the homepage by Community♦ 3 hours ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
up vote
4
down vote
favorite
up vote
4
down vote
favorite
I have a Symfony2 project. I have an Entity Asset
which can have relations with Category
. I store a categoryCount
-field within the Asset
to determine if an asset has one, to select those fast via a DQL-query depending on the current user state.
Now found myself writing something along the lines of this in my AssetRepository
:
/**
* @param string $orderBy
* @param int $mode
*
* @return DoctrineORMQuery
*/
public function getQueryForAll($orderBy = 'DESC', $mode = self::FILTER_WITHOUT_QUARANTINED)
{
$qb = $this->createQueryBuilder('asset');
$qb->orderBy('asset.updatedAt', $orderBy);
$filter = $this->getQuarantinedCriteria($mode);
if ($filter) {
$qb->addCriteria($filter);
}
return $query = $qb->getQuery();
}
/**
* @param int $mode
*
* @return Criteria|null
*/
protected function getQuarantinedCriteria($mode = self::FILTER_WITHOUT_QUARANTINED) {
$filter = null;
if ($mode === self::FILTER_WITHOUT_QUARANTINED) {
$filter = Criteria::create()
->andWhere(Criteria::expr()->gt('categoryCount', 0));
} else if ($mode === self::FILTER_ONLY_QURANTINED) {
$filter = Criteria::create()
->andWhere(Criteria::expr()->eq('categoryCount', 0));
}
return $filter;
}
This doesn't feel right to me. The $mode
flag triggers my code smell alarm, I also am worrying about passing it into each asset querying method.
Furthermore, since I will have to set the mode depending on the state of the current logged in user, I also am worried that I will produce code duplications by doing a lot of if-user-has-the-rights-checks in my actions or services:
/**
* @Route("/all_assets/{page}",
* name="get_paginated_assets",
* requirements={"page" = "d+"},
* defaults={"page" = "1"},
* options={"expose"=true}
* )
*/
public function getPaginatedAssetActions($page)
{
$repo = $this->getAssetRepository();
$isAllowedToViewRestrictedAssets = $this->getCurrentUser()->isAdmin();
if ($isAllowedToViewRestrictedAssets) {
$mode = EntityAssetRepository::FILTER_SHOW_ALL;
}
$query = $repo->getQueryForAll($mode);
$pagerfanta = new Pagerfanta(new DoctrineORMAdapter($query));
$data = $this->getCurrentPagedResults($pagerfanta, $page);
$view = $this->view();
$view->setFormat('json');
$view->setData($data);
return $view;
}
Is this the right way to implement the logic to enable filters in a Repository? Feedback on whether there is a more decoupled way would be highly appreciated.
php php5 doctrine
I have a Symfony2 project. I have an Entity Asset
which can have relations with Category
. I store a categoryCount
-field within the Asset
to determine if an asset has one, to select those fast via a DQL-query depending on the current user state.
Now found myself writing something along the lines of this in my AssetRepository
:
/**
* @param string $orderBy
* @param int $mode
*
* @return DoctrineORMQuery
*/
public function getQueryForAll($orderBy = 'DESC', $mode = self::FILTER_WITHOUT_QUARANTINED)
{
$qb = $this->createQueryBuilder('asset');
$qb->orderBy('asset.updatedAt', $orderBy);
$filter = $this->getQuarantinedCriteria($mode);
if ($filter) {
$qb->addCriteria($filter);
}
return $query = $qb->getQuery();
}
/**
* @param int $mode
*
* @return Criteria|null
*/
protected function getQuarantinedCriteria($mode = self::FILTER_WITHOUT_QUARANTINED) {
$filter = null;
if ($mode === self::FILTER_WITHOUT_QUARANTINED) {
$filter = Criteria::create()
->andWhere(Criteria::expr()->gt('categoryCount', 0));
} else if ($mode === self::FILTER_ONLY_QURANTINED) {
$filter = Criteria::create()
->andWhere(Criteria::expr()->eq('categoryCount', 0));
}
return $filter;
}
This doesn't feel right to me. The $mode
flag triggers my code smell alarm, I also am worrying about passing it into each asset querying method.
Furthermore, since I will have to set the mode depending on the state of the current logged in user, I also am worried that I will produce code duplications by doing a lot of if-user-has-the-rights-checks in my actions or services:
/**
* @Route("/all_assets/{page}",
* name="get_paginated_assets",
* requirements={"page" = "d+"},
* defaults={"page" = "1"},
* options={"expose"=true}
* )
*/
public function getPaginatedAssetActions($page)
{
$repo = $this->getAssetRepository();
$isAllowedToViewRestrictedAssets = $this->getCurrentUser()->isAdmin();
if ($isAllowedToViewRestrictedAssets) {
$mode = EntityAssetRepository::FILTER_SHOW_ALL;
}
$query = $repo->getQueryForAll($mode);
$pagerfanta = new Pagerfanta(new DoctrineORMAdapter($query));
$data = $this->getCurrentPagedResults($pagerfanta, $page);
$view = $this->view();
$view->setFormat('json');
$view->setData($data);
return $view;
}
Is this the right way to implement the logic to enable filters in a Repository? Feedback on whether there is a more decoupled way would be highly appreciated.
php php5 doctrine
php php5 doctrine
edited Apr 17 '14 at 11:32
rolfl♦
90.6k13190393
90.6k13190393
asked Apr 17 '14 at 10:46


k0pernikus
1538
1538
bumped to the homepage by Community♦ 3 hours ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
bumped to the homepage by Community♦ 3 hours ago
This question has answers that may be good or bad; the system has marked it active so that they can be reviewed.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You can try to use SQLFilter for this purpose. It allows you to tune SQL query based on some logic. You can pass information about your user's state into filter through DI container and use this information to tune your SQL query. Please note that unlike repositories - SQLFilter works on SQL level, not on DQL. More information can be found into Doctrine's documentation.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You can try to use SQLFilter for this purpose. It allows you to tune SQL query based on some logic. You can pass information about your user's state into filter through DI container and use this information to tune your SQL query. Please note that unlike repositories - SQLFilter works on SQL level, not on DQL. More information can be found into Doctrine's documentation.
add a comment |
up vote
0
down vote
You can try to use SQLFilter for this purpose. It allows you to tune SQL query based on some logic. You can pass information about your user's state into filter through DI container and use this information to tune your SQL query. Please note that unlike repositories - SQLFilter works on SQL level, not on DQL. More information can be found into Doctrine's documentation.
add a comment |
up vote
0
down vote
up vote
0
down vote
You can try to use SQLFilter for this purpose. It allows you to tune SQL query based on some logic. You can pass information about your user's state into filter through DI container and use this information to tune your SQL query. Please note that unlike repositories - SQLFilter works on SQL level, not on DQL. More information can be found into Doctrine's documentation.
You can try to use SQLFilter for this purpose. It allows you to tune SQL query based on some logic. You can pass information about your user's state into filter through DI container and use this information to tune your SQL query. Please note that unlike repositories - SQLFilter works on SQL level, not on DQL. More information can be found into Doctrine's documentation.
answered May 21 '17 at 21:14
Flying
21123
21123
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f47455%2ffiltering-queries-based-on-the-current-user-state%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
t jZTDCnXjk,BjKfQidUvd,0dVu8SW1TiEmfTUlYYHSLsnTOCwX3Ojh5 nl wy0OlzRqt Q