React + Django (session) authentication
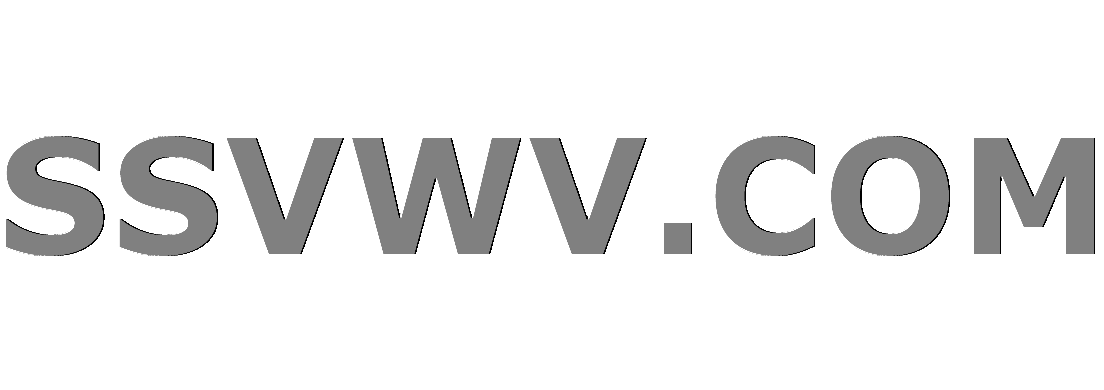
Multi tool use
up vote
1
down vote
favorite
Is authentication implemented correctly?
At the entry point to the app, which is App.js
, query the Django server, which responds whether the current user is authenticated after checking request.user.isAuthenticated
I am not using redux
, just storing authentication state in a local object (this can't be tampered with I assume?). This is reset on signout and I guess it is also lost and re-queried whenever the app is reloaded.
All app routes except the login route are behind PrivateRoute
, which will redirect to login unless myAuth.isAuthenticated === true
.
Any additional comments welcome.
App.js
import React, {Component} from "react";
import ReactDOM from "react-dom";
import MainContainer from "../containers/MainContainer";
import {Provider} from "react-redux";
import store from "../store";
import BrowserRouter from "react-router-dom/es/BrowserRouter";
import {Redirect, Route, Switch} from "react-router-dom";
import LoginPage from "./LoginPage";
import myAuth from "../myAuth";
import {APP_LOGIN_PATH} from "../constants/paths";
class App extends Component {
state = {
loaded: false,
};
componentDidMount() {
myAuth.authenticate(() => this.setState({
loaded: true,
}))
}
render() {
const { loaded } = this.state;
return (
loaded ? (
<BrowserRouter>
<Switch>
<Route path={ APP_LOGIN_PATH } component={ LoginPage } />
<PrivateRoute path="/app" component={ MainContainer } />
</Switch>
</BrowserRouter>
) : (
<div>Loading...</div>
)
)
}
}
const PrivateRoute = ({ component: Component, ...rest }) => (
<Route {...rest} render={(props) => (
myAuth.isAuthenticated === true
? <Component {...props} />
: <Redirect to={{ pathname: APP_LOGIN_PATH, state: { from: props.location }}} />
)} />
);
const wrapper = document.getElementById("app");
wrapper ? ReactDOM.render(
<Provider store={ store }>
<BrowserRouter>
<App />
</BrowserRouter>
</Provider>
, wrapper) : null;
myAuth.js
const myAuth = {
isAuthenticated: false,
isStaff: false,
isSuperuser: false,
authenticate(cb) {
console.log("authenticate");
fetch("/session_user/")
.then(response => {
if (response.status !== 200) {
console.log("Something went wrong during authentication");
}
return response.json();
})
.then(data => {
this.isAuthenticated = data.isAuthenticated;
this.isStaff = data.isStaff;
this.isSuperuser = data.isSuperuser;
})
.then(cb)
},
signout(cb) {
fetch("/logout/")
.then(response => {
if (response.status !== 200) {
console.log("Something went wrong while logging out");
}
})
.then(data => {
this.isAuthenticated = false;
this.isStaff = false;
this.isSuperuser = false;
})
}
};
export default myAuth;
authentication react.js django jsx
add a comment |
up vote
1
down vote
favorite
Is authentication implemented correctly?
At the entry point to the app, which is App.js
, query the Django server, which responds whether the current user is authenticated after checking request.user.isAuthenticated
I am not using redux
, just storing authentication state in a local object (this can't be tampered with I assume?). This is reset on signout and I guess it is also lost and re-queried whenever the app is reloaded.
All app routes except the login route are behind PrivateRoute
, which will redirect to login unless myAuth.isAuthenticated === true
.
Any additional comments welcome.
App.js
import React, {Component} from "react";
import ReactDOM from "react-dom";
import MainContainer from "../containers/MainContainer";
import {Provider} from "react-redux";
import store from "../store";
import BrowserRouter from "react-router-dom/es/BrowserRouter";
import {Redirect, Route, Switch} from "react-router-dom";
import LoginPage from "./LoginPage";
import myAuth from "../myAuth";
import {APP_LOGIN_PATH} from "../constants/paths";
class App extends Component {
state = {
loaded: false,
};
componentDidMount() {
myAuth.authenticate(() => this.setState({
loaded: true,
}))
}
render() {
const { loaded } = this.state;
return (
loaded ? (
<BrowserRouter>
<Switch>
<Route path={ APP_LOGIN_PATH } component={ LoginPage } />
<PrivateRoute path="/app" component={ MainContainer } />
</Switch>
</BrowserRouter>
) : (
<div>Loading...</div>
)
)
}
}
const PrivateRoute = ({ component: Component, ...rest }) => (
<Route {...rest} render={(props) => (
myAuth.isAuthenticated === true
? <Component {...props} />
: <Redirect to={{ pathname: APP_LOGIN_PATH, state: { from: props.location }}} />
)} />
);
const wrapper = document.getElementById("app");
wrapper ? ReactDOM.render(
<Provider store={ store }>
<BrowserRouter>
<App />
</BrowserRouter>
</Provider>
, wrapper) : null;
myAuth.js
const myAuth = {
isAuthenticated: false,
isStaff: false,
isSuperuser: false,
authenticate(cb) {
console.log("authenticate");
fetch("/session_user/")
.then(response => {
if (response.status !== 200) {
console.log("Something went wrong during authentication");
}
return response.json();
})
.then(data => {
this.isAuthenticated = data.isAuthenticated;
this.isStaff = data.isStaff;
this.isSuperuser = data.isSuperuser;
})
.then(cb)
},
signout(cb) {
fetch("/logout/")
.then(response => {
if (response.status !== 200) {
console.log("Something went wrong while logging out");
}
})
.then(data => {
this.isAuthenticated = false;
this.isStaff = false;
this.isSuperuser = false;
})
}
};
export default myAuth;
authentication react.js django jsx
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Is authentication implemented correctly?
At the entry point to the app, which is App.js
, query the Django server, which responds whether the current user is authenticated after checking request.user.isAuthenticated
I am not using redux
, just storing authentication state in a local object (this can't be tampered with I assume?). This is reset on signout and I guess it is also lost and re-queried whenever the app is reloaded.
All app routes except the login route are behind PrivateRoute
, which will redirect to login unless myAuth.isAuthenticated === true
.
Any additional comments welcome.
App.js
import React, {Component} from "react";
import ReactDOM from "react-dom";
import MainContainer from "../containers/MainContainer";
import {Provider} from "react-redux";
import store from "../store";
import BrowserRouter from "react-router-dom/es/BrowserRouter";
import {Redirect, Route, Switch} from "react-router-dom";
import LoginPage from "./LoginPage";
import myAuth from "../myAuth";
import {APP_LOGIN_PATH} from "../constants/paths";
class App extends Component {
state = {
loaded: false,
};
componentDidMount() {
myAuth.authenticate(() => this.setState({
loaded: true,
}))
}
render() {
const { loaded } = this.state;
return (
loaded ? (
<BrowserRouter>
<Switch>
<Route path={ APP_LOGIN_PATH } component={ LoginPage } />
<PrivateRoute path="/app" component={ MainContainer } />
</Switch>
</BrowserRouter>
) : (
<div>Loading...</div>
)
)
}
}
const PrivateRoute = ({ component: Component, ...rest }) => (
<Route {...rest} render={(props) => (
myAuth.isAuthenticated === true
? <Component {...props} />
: <Redirect to={{ pathname: APP_LOGIN_PATH, state: { from: props.location }}} />
)} />
);
const wrapper = document.getElementById("app");
wrapper ? ReactDOM.render(
<Provider store={ store }>
<BrowserRouter>
<App />
</BrowserRouter>
</Provider>
, wrapper) : null;
myAuth.js
const myAuth = {
isAuthenticated: false,
isStaff: false,
isSuperuser: false,
authenticate(cb) {
console.log("authenticate");
fetch("/session_user/")
.then(response => {
if (response.status !== 200) {
console.log("Something went wrong during authentication");
}
return response.json();
})
.then(data => {
this.isAuthenticated = data.isAuthenticated;
this.isStaff = data.isStaff;
this.isSuperuser = data.isSuperuser;
})
.then(cb)
},
signout(cb) {
fetch("/logout/")
.then(response => {
if (response.status !== 200) {
console.log("Something went wrong while logging out");
}
})
.then(data => {
this.isAuthenticated = false;
this.isStaff = false;
this.isSuperuser = false;
})
}
};
export default myAuth;
authentication react.js django jsx
Is authentication implemented correctly?
At the entry point to the app, which is App.js
, query the Django server, which responds whether the current user is authenticated after checking request.user.isAuthenticated
I am not using redux
, just storing authentication state in a local object (this can't be tampered with I assume?). This is reset on signout and I guess it is also lost and re-queried whenever the app is reloaded.
All app routes except the login route are behind PrivateRoute
, which will redirect to login unless myAuth.isAuthenticated === true
.
Any additional comments welcome.
App.js
import React, {Component} from "react";
import ReactDOM from "react-dom";
import MainContainer from "../containers/MainContainer";
import {Provider} from "react-redux";
import store from "../store";
import BrowserRouter from "react-router-dom/es/BrowserRouter";
import {Redirect, Route, Switch} from "react-router-dom";
import LoginPage from "./LoginPage";
import myAuth from "../myAuth";
import {APP_LOGIN_PATH} from "../constants/paths";
class App extends Component {
state = {
loaded: false,
};
componentDidMount() {
myAuth.authenticate(() => this.setState({
loaded: true,
}))
}
render() {
const { loaded } = this.state;
return (
loaded ? (
<BrowserRouter>
<Switch>
<Route path={ APP_LOGIN_PATH } component={ LoginPage } />
<PrivateRoute path="/app" component={ MainContainer } />
</Switch>
</BrowserRouter>
) : (
<div>Loading...</div>
)
)
}
}
const PrivateRoute = ({ component: Component, ...rest }) => (
<Route {...rest} render={(props) => (
myAuth.isAuthenticated === true
? <Component {...props} />
: <Redirect to={{ pathname: APP_LOGIN_PATH, state: { from: props.location }}} />
)} />
);
const wrapper = document.getElementById("app");
wrapper ? ReactDOM.render(
<Provider store={ store }>
<BrowserRouter>
<App />
</BrowserRouter>
</Provider>
, wrapper) : null;
myAuth.js
const myAuth = {
isAuthenticated: false,
isStaff: false,
isSuperuser: false,
authenticate(cb) {
console.log("authenticate");
fetch("/session_user/")
.then(response => {
if (response.status !== 200) {
console.log("Something went wrong during authentication");
}
return response.json();
})
.then(data => {
this.isAuthenticated = data.isAuthenticated;
this.isStaff = data.isStaff;
this.isSuperuser = data.isSuperuser;
})
.then(cb)
},
signout(cb) {
fetch("/logout/")
.then(response => {
if (response.status !== 200) {
console.log("Something went wrong while logging out");
}
})
.then(data => {
this.isAuthenticated = false;
this.isStaff = false;
this.isSuperuser = false;
})
}
};
export default myAuth;
authentication react.js django jsx
authentication react.js django jsx
edited 2 days ago


200_success
127k15148410
127k15148410
asked 2 days ago
M3RS
1304
1304
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f207485%2freact-django-session-authentication%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Y QBLOw5a0WKlewL8hki4bq ACwK22LMBm064lem pT 7yYqn WHfb7H1BJ TS,xnYW