Calculate your Icy Tower Score
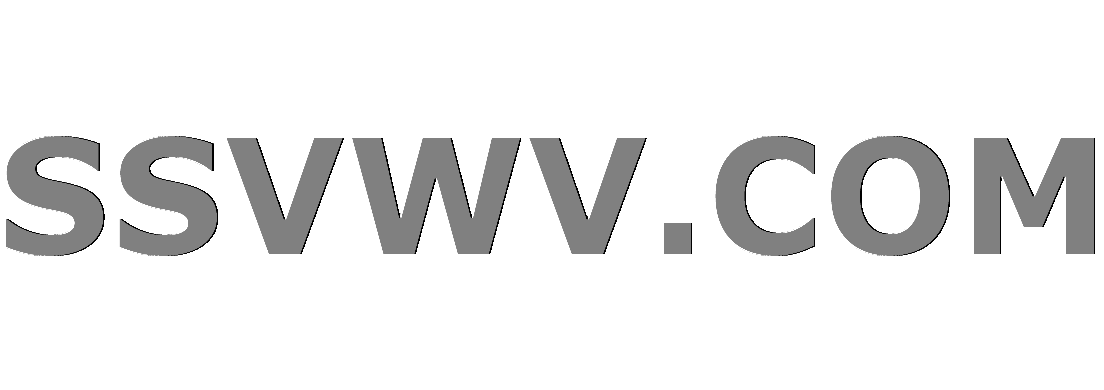
Multi tool use
up vote
6
down vote
favorite
Do you know Icy Tower ? Here is a gameplay example of what i'm talking about
The game concept is simple : you play as Harold the Homeboy, and your goal is to jump from floors to floors to go as high as possible without falling and getting off-screen ( the camera goes higher following you or at a speed that gets faster over time, and does not go down ).
If you get off-screen ( fell or camera was too fast for you to keep up ), it's game over, and then you get a score.
Your task is to write a program/function/whatever that calculates the score based on a input.
How to calculate the score :
The formula is simple : $Score = 10 * LastFloor + Sum(ValidComboList.TotalFloorJumped^2)$
What is a valid combo ?
You have to jump from floors to floors to go as high as possible. Sometimes, you will the to the floor $F+1$
But sometimes, if you go fast enough ( faster = higher jumps ), you will do a multi-floor jump, and skip some floors between. A combo is a sequence of multi-floors jump. To be considered as a multi-floor jump, you need to skip at least 1 floor, meaning you need to get to the floor $F+x$ where $x >= 2$
See that you always jump to $x >= 2$, jumps to lower floors with floors skipped between are not considered valid
A combo ends when :
- You jump to the floor $F+x$ where $(x < 0) | (x == 1)$
- You wait more than about 3 seconds on the same floor ( in the gameplay video, you can see on the left a "timing bar", and the combo ends if it gets empty )
- You get off-screen ( falling or not jumping fast enough )
In this challenge, to simplify a bit, we will consider that, when the input indicates a "jump to the same floor $F+0$" means that the 3 second timeout is passed, so the two rules will be simplified in one :
"You jump to the floor $F+x$ where $x<2$
A valid combo ( a combo that will be used for the score calculation ) :
- The number of multi-floor jumps $N >= 2$
- The combo MUST NOT end with going off-screen. If you go off-screen, the combo is invalid and won't included in the score. Said in another way : The combo must be followed by a jump to another floor or by waiting about 3 seconds.
In this challenge, since we consider that jumping to the same floor is waiting 3 seconds, the rules to make a valid combo is "You must jump to another floor"
Since you need at least 2 multi-floor jumps to make a combo, the number of total floor jumped is higher than 4
The input
The input will be a "list" of integers ( or STDIN input ) which represents the sequence of floor jumped on. For example, the start to the video linked previously in the post will look like :
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 54]
for the first floors. The start floor is ommited. This input end on floor 54 meaning he went off-screen just after that, without getting to another floor, so there's the game over ( this was not the case in the video since he went to the floor 232, so the array accurately representing the sequence would end with 232 at the end )
Here, with this input, you must output $829$, because :
$Score = 10 * 54 + 12^2 + 8^2 + 9^2$ Because the last floor Harold was on is the floor number 54, and he made 3 valid combos :
- First combo of 4 multi-floor jumps with a total of 12 floors jumped in that combo
- Second combo of 2 multi-floor jumps with a total of 8 floors jumped in that combo
- The last combo of 2 multi-floor jumps with a total of 9 floors jumped in that combo
Test cases :
=> Undefined Behavior
[5]
=> $50$
[4 0 5 6 8 10 10]
=> $10*10 + 4^2 = 116$ Correction applied
[4 0 5 6 6 6 6 8 10 10]
=> $10*10 + 4^2 = 116$ ( Here, Harold just waited 9 seconds. No combo to validate, but still a legitimate input ) Correction applied
[5 7 10 10 11 9 14 17 15 16]
=> $10*16 + 10^2 + 8^2 = 324$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 54]
=> $10 * 54 + 12^2 + 8^2 + 9^2 = 829$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 56]
=> $10 * 54 + 12^2 + 8^2 + 9^2 = 849$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 55]
=> $10 * 55 + 12^2 + 8^2 + 9^2 = 839$ ( harold waited 3 seconds to validate the combo )
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55]
=> $10 * 55 + 12^2 + 8^2 = 758$ ( because harold went off-screen without confirming the combo)
If you want, you can condsider that the input always starts with zero ( since the game start at floor 0 ), if this is the case, say it in your answer
Rules :
- Input and output in any convenient way
- Full program, or function, or lambda, or macro are acceptable
Standard Loopholes are forbidden- This is code-golf, so lower number of bytes are better
Edit : Correction applied on some test cases
code-golf
add a comment |
up vote
6
down vote
favorite
Do you know Icy Tower ? Here is a gameplay example of what i'm talking about
The game concept is simple : you play as Harold the Homeboy, and your goal is to jump from floors to floors to go as high as possible without falling and getting off-screen ( the camera goes higher following you or at a speed that gets faster over time, and does not go down ).
If you get off-screen ( fell or camera was too fast for you to keep up ), it's game over, and then you get a score.
Your task is to write a program/function/whatever that calculates the score based on a input.
How to calculate the score :
The formula is simple : $Score = 10 * LastFloor + Sum(ValidComboList.TotalFloorJumped^2)$
What is a valid combo ?
You have to jump from floors to floors to go as high as possible. Sometimes, you will the to the floor $F+1$
But sometimes, if you go fast enough ( faster = higher jumps ), you will do a multi-floor jump, and skip some floors between. A combo is a sequence of multi-floors jump. To be considered as a multi-floor jump, you need to skip at least 1 floor, meaning you need to get to the floor $F+x$ where $x >= 2$
See that you always jump to $x >= 2$, jumps to lower floors with floors skipped between are not considered valid
A combo ends when :
- You jump to the floor $F+x$ where $(x < 0) | (x == 1)$
- You wait more than about 3 seconds on the same floor ( in the gameplay video, you can see on the left a "timing bar", and the combo ends if it gets empty )
- You get off-screen ( falling or not jumping fast enough )
In this challenge, to simplify a bit, we will consider that, when the input indicates a "jump to the same floor $F+0$" means that the 3 second timeout is passed, so the two rules will be simplified in one :
"You jump to the floor $F+x$ where $x<2$
A valid combo ( a combo that will be used for the score calculation ) :
- The number of multi-floor jumps $N >= 2$
- The combo MUST NOT end with going off-screen. If you go off-screen, the combo is invalid and won't included in the score. Said in another way : The combo must be followed by a jump to another floor or by waiting about 3 seconds.
In this challenge, since we consider that jumping to the same floor is waiting 3 seconds, the rules to make a valid combo is "You must jump to another floor"
Since you need at least 2 multi-floor jumps to make a combo, the number of total floor jumped is higher than 4
The input
The input will be a "list" of integers ( or STDIN input ) which represents the sequence of floor jumped on. For example, the start to the video linked previously in the post will look like :
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 54]
for the first floors. The start floor is ommited. This input end on floor 54 meaning he went off-screen just after that, without getting to another floor, so there's the game over ( this was not the case in the video since he went to the floor 232, so the array accurately representing the sequence would end with 232 at the end )
Here, with this input, you must output $829$, because :
$Score = 10 * 54 + 12^2 + 8^2 + 9^2$ Because the last floor Harold was on is the floor number 54, and he made 3 valid combos :
- First combo of 4 multi-floor jumps with a total of 12 floors jumped in that combo
- Second combo of 2 multi-floor jumps with a total of 8 floors jumped in that combo
- The last combo of 2 multi-floor jumps with a total of 9 floors jumped in that combo
Test cases :
=> Undefined Behavior
[5]
=> $50$
[4 0 5 6 8 10 10]
=> $10*10 + 4^2 = 116$ Correction applied
[4 0 5 6 6 6 6 8 10 10]
=> $10*10 + 4^2 = 116$ ( Here, Harold just waited 9 seconds. No combo to validate, but still a legitimate input ) Correction applied
[5 7 10 10 11 9 14 17 15 16]
=> $10*16 + 10^2 + 8^2 = 324$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 54]
=> $10 * 54 + 12^2 + 8^2 + 9^2 = 829$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 56]
=> $10 * 54 + 12^2 + 8^2 + 9^2 = 849$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 55]
=> $10 * 55 + 12^2 + 8^2 + 9^2 = 839$ ( harold waited 3 seconds to validate the combo )
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55]
=> $10 * 55 + 12^2 + 8^2 = 758$ ( because harold went off-screen without confirming the combo)
If you want, you can condsider that the input always starts with zero ( since the game start at floor 0 ), if this is the case, say it in your answer
Rules :
- Input and output in any convenient way
- Full program, or function, or lambda, or macro are acceptable
Standard Loopholes are forbidden- This is code-golf, so lower number of bytes are better
Edit : Correction applied on some test cases
code-golf
add a comment |
up vote
6
down vote
favorite
up vote
6
down vote
favorite
Do you know Icy Tower ? Here is a gameplay example of what i'm talking about
The game concept is simple : you play as Harold the Homeboy, and your goal is to jump from floors to floors to go as high as possible without falling and getting off-screen ( the camera goes higher following you or at a speed that gets faster over time, and does not go down ).
If you get off-screen ( fell or camera was too fast for you to keep up ), it's game over, and then you get a score.
Your task is to write a program/function/whatever that calculates the score based on a input.
How to calculate the score :
The formula is simple : $Score = 10 * LastFloor + Sum(ValidComboList.TotalFloorJumped^2)$
What is a valid combo ?
You have to jump from floors to floors to go as high as possible. Sometimes, you will the to the floor $F+1$
But sometimes, if you go fast enough ( faster = higher jumps ), you will do a multi-floor jump, and skip some floors between. A combo is a sequence of multi-floors jump. To be considered as a multi-floor jump, you need to skip at least 1 floor, meaning you need to get to the floor $F+x$ where $x >= 2$
See that you always jump to $x >= 2$, jumps to lower floors with floors skipped between are not considered valid
A combo ends when :
- You jump to the floor $F+x$ where $(x < 0) | (x == 1)$
- You wait more than about 3 seconds on the same floor ( in the gameplay video, you can see on the left a "timing bar", and the combo ends if it gets empty )
- You get off-screen ( falling or not jumping fast enough )
In this challenge, to simplify a bit, we will consider that, when the input indicates a "jump to the same floor $F+0$" means that the 3 second timeout is passed, so the two rules will be simplified in one :
"You jump to the floor $F+x$ where $x<2$
A valid combo ( a combo that will be used for the score calculation ) :
- The number of multi-floor jumps $N >= 2$
- The combo MUST NOT end with going off-screen. If you go off-screen, the combo is invalid and won't included in the score. Said in another way : The combo must be followed by a jump to another floor or by waiting about 3 seconds.
In this challenge, since we consider that jumping to the same floor is waiting 3 seconds, the rules to make a valid combo is "You must jump to another floor"
Since you need at least 2 multi-floor jumps to make a combo, the number of total floor jumped is higher than 4
The input
The input will be a "list" of integers ( or STDIN input ) which represents the sequence of floor jumped on. For example, the start to the video linked previously in the post will look like :
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 54]
for the first floors. The start floor is ommited. This input end on floor 54 meaning he went off-screen just after that, without getting to another floor, so there's the game over ( this was not the case in the video since he went to the floor 232, so the array accurately representing the sequence would end with 232 at the end )
Here, with this input, you must output $829$, because :
$Score = 10 * 54 + 12^2 + 8^2 + 9^2$ Because the last floor Harold was on is the floor number 54, and he made 3 valid combos :
- First combo of 4 multi-floor jumps with a total of 12 floors jumped in that combo
- Second combo of 2 multi-floor jumps with a total of 8 floors jumped in that combo
- The last combo of 2 multi-floor jumps with a total of 9 floors jumped in that combo
Test cases :
=> Undefined Behavior
[5]
=> $50$
[4 0 5 6 8 10 10]
=> $10*10 + 4^2 = 116$ Correction applied
[4 0 5 6 6 6 6 8 10 10]
=> $10*10 + 4^2 = 116$ ( Here, Harold just waited 9 seconds. No combo to validate, but still a legitimate input ) Correction applied
[5 7 10 10 11 9 14 17 15 16]
=> $10*16 + 10^2 + 8^2 = 324$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 54]
=> $10 * 54 + 12^2 + 8^2 + 9^2 = 829$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 56]
=> $10 * 54 + 12^2 + 8^2 + 9^2 = 849$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 55]
=> $10 * 55 + 12^2 + 8^2 + 9^2 = 839$ ( harold waited 3 seconds to validate the combo )
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55]
=> $10 * 55 + 12^2 + 8^2 = 758$ ( because harold went off-screen without confirming the combo)
If you want, you can condsider that the input always starts with zero ( since the game start at floor 0 ), if this is the case, say it in your answer
Rules :
- Input and output in any convenient way
- Full program, or function, or lambda, or macro are acceptable
Standard Loopholes are forbidden- This is code-golf, so lower number of bytes are better
Edit : Correction applied on some test cases
code-golf
Do you know Icy Tower ? Here is a gameplay example of what i'm talking about
The game concept is simple : you play as Harold the Homeboy, and your goal is to jump from floors to floors to go as high as possible without falling and getting off-screen ( the camera goes higher following you or at a speed that gets faster over time, and does not go down ).
If you get off-screen ( fell or camera was too fast for you to keep up ), it's game over, and then you get a score.
Your task is to write a program/function/whatever that calculates the score based on a input.
How to calculate the score :
The formula is simple : $Score = 10 * LastFloor + Sum(ValidComboList.TotalFloorJumped^2)$
What is a valid combo ?
You have to jump from floors to floors to go as high as possible. Sometimes, you will the to the floor $F+1$
But sometimes, if you go fast enough ( faster = higher jumps ), you will do a multi-floor jump, and skip some floors between. A combo is a sequence of multi-floors jump. To be considered as a multi-floor jump, you need to skip at least 1 floor, meaning you need to get to the floor $F+x$ where $x >= 2$
See that you always jump to $x >= 2$, jumps to lower floors with floors skipped between are not considered valid
A combo ends when :
- You jump to the floor $F+x$ where $(x < 0) | (x == 1)$
- You wait more than about 3 seconds on the same floor ( in the gameplay video, you can see on the left a "timing bar", and the combo ends if it gets empty )
- You get off-screen ( falling or not jumping fast enough )
In this challenge, to simplify a bit, we will consider that, when the input indicates a "jump to the same floor $F+0$" means that the 3 second timeout is passed, so the two rules will be simplified in one :
"You jump to the floor $F+x$ where $x<2$
A valid combo ( a combo that will be used for the score calculation ) :
- The number of multi-floor jumps $N >= 2$
- The combo MUST NOT end with going off-screen. If you go off-screen, the combo is invalid and won't included in the score. Said in another way : The combo must be followed by a jump to another floor or by waiting about 3 seconds.
In this challenge, since we consider that jumping to the same floor is waiting 3 seconds, the rules to make a valid combo is "You must jump to another floor"
Since you need at least 2 multi-floor jumps to make a combo, the number of total floor jumped is higher than 4
The input
The input will be a "list" of integers ( or STDIN input ) which represents the sequence of floor jumped on. For example, the start to the video linked previously in the post will look like :
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 54]
for the first floors. The start floor is ommited. This input end on floor 54 meaning he went off-screen just after that, without getting to another floor, so there's the game over ( this was not the case in the video since he went to the floor 232, so the array accurately representing the sequence would end with 232 at the end )
Here, with this input, you must output $829$, because :
$Score = 10 * 54 + 12^2 + 8^2 + 9^2$ Because the last floor Harold was on is the floor number 54, and he made 3 valid combos :
- First combo of 4 multi-floor jumps with a total of 12 floors jumped in that combo
- Second combo of 2 multi-floor jumps with a total of 8 floors jumped in that combo
- The last combo of 2 multi-floor jumps with a total of 9 floors jumped in that combo
Test cases :
=> Undefined Behavior
[5]
=> $50$
[4 0 5 6 8 10 10]
=> $10*10 + 4^2 = 116$ Correction applied
[4 0 5 6 6 6 6 8 10 10]
=> $10*10 + 4^2 = 116$ ( Here, Harold just waited 9 seconds. No combo to validate, but still a legitimate input ) Correction applied
[5 7 10 10 11 9 14 17 15 16]
=> $10*16 + 10^2 + 8^2 = 324$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 54]
=> $10 * 54 + 12^2 + 8^2 + 9^2 = 829$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 56]
=> $10 * 54 + 12^2 + 8^2 + 9^2 = 849$
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55 55]
=> $10 * 55 + 12^2 + 8^2 + 9^2 = 839$ ( harold waited 3 seconds to validate the combo )
[5 4 5 10 12 15 17 18 19 20 23 24 25 28 29 30 31 34 35 36 37 34 35 36 37 38 39 40 43 48 45 46 50 55]
=> $10 * 55 + 12^2 + 8^2 = 758$ ( because harold went off-screen without confirming the combo)
If you want, you can condsider that the input always starts with zero ( since the game start at floor 0 ), if this is the case, say it in your answer
Rules :
- Input and output in any convenient way
- Full program, or function, or lambda, or macro are acceptable
Standard Loopholes are forbidden- This is code-golf, so lower number of bytes are better
Edit : Correction applied on some test cases
code-golf
code-golf
edited Nov 13 at 19:35
asked Nov 13 at 18:15


HatsuPointerKun
1,7511211
1,7511211
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
up vote
5
down vote
Japt, 28 27 24 bytes
-1 byte from @kamil <3, -3 from @Shaggy :o. These guys are genius
ä- ô>2n)¯J lÉ mx x²+A*Uo
Try it online! or Test it all
Japt, 28 bytes, prev approach
Naive Way.
Important Input should start with 0 to work correctly (in some cases)
o *A+UóÈĦY©Y>XÃl>2 ®ä- xÃxp
Try it online! or Test it all
1
27 bytes
– Kamil Drakari
Nov 13 at 21:31
1
Quick 3 byte saving
– Shaggy
Nov 14 at 0:04
add a comment |
up vote
2
down vote
JavaScript (ES7), 72 bytes
a=>a.map(v=>(v-p>1?r=c++?r:p:t+=(c>1)*(c=0,p-r)**2,p=v),t=c=p=0)&&t+p*10
Try it online!
Commented
a => // a = input array
a.map(v => ( // for each value v in a:
v - p > 1 ? // if the difference with the previous floor is greater than 1:
r = c++ ? // increment the combo counter c; if c was not equal to 0:
r // leave r unchanged
: // else:
p // set r (reference floor) to the previous floor p
: // else:
t += // update t only ...
(c > 1) * ( // ... if we have a multi-floor jump combo of at least 2 jumps
c = 0, // reset c to 0
p - r // compute the total number of floors jumped in the combo
) ** 2, // square it and add it to t
p = v // set the previous value p to v
), //
t = c = p = 0 // start with t = c = p = 0
) && // end of map()
t + p * 10 // add the final floor multiplied by 10 to t
add a comment |
up vote
2
down vote
J, 54 bytes
[:((10*{:)+1#.[:}:]((2<#)*[:*:{:-{.);.1~1,2>2-~/])0&,
Try it online!
May add explanation later. For now here's an ungolfed and a parsed version:
ungolfed
[: ((10 * {:) + 1 #. [: }: ] ((2 < #) * [: *: {: - {.);.1~ 1 , 2 > 2 -~/ ]) 0&,
parsed
┌──┬─────────────────────────────────────────────────────────────────────────────────────────────────────────┬───────┐
│[:│┌─────────┬─┬───────────────────────────────────────────────────────────────────────────────────────────┐│┌─┬─┬─┐│
│ ││┌──┬─┬──┐│+│┌─┬──┬────────────────────────────────────────────────────────────────────────────────────┐│││0│&│,││
│ │││10│*│{:││ ││1│#.│┌──┬──┬────────────────────────────────────────────────────────────────────────────┐│││└─┴─┴─┘│
│ ││└──┴─┴──┘│ ││ │ ││[:│}:│┌─┬────────────────────────────────────────┬───────────────────────────────┐││││ │
│ ││ │ ││ │ ││ │ ││]│┌────────────────────────────────────┬─┐│┌─┬─┬─────────────────────────┐│││││ │
│ ││ │ ││ │ ││ │ ││ ││┌─────────────────────────────┬──┬─┐│~│││1│,│┌─┬─┬───────────────────┐││││││ │
│ ││ │ ││ │ ││ │ ││ │││┌───────┬─┬─────────────────┐│;.│1││ │││ │ ││2│>│┌─┬─────────────┬─┐│││││││ │
│ ││ │ ││ │ ││ │ ││ ││││┌─┬─┬─┐│*│┌──┬──┬─────────┐││ │ ││ │││ │ ││ │ ││2│┌─────────┬─┐│]││││││││ │
│ ││ │ ││ │ ││ │ ││ │││││2│<│#││ ││[:│*:│┌──┬─┬──┐│││ │ ││ │││ │ ││ │ ││ ││┌─────┬─┐│││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││└─┴─┴─┘│ ││ │ ││{:│-│{.││││ │ ││ │││ │ ││ │ ││ │││┌─┬─┐│/││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ ││ │ │└──┴─┴──┘│││ │ ││ │││ │ ││ │ ││ ││││-│~││ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ │└──┴──┴─────────┘││ │ ││ │││ │ ││ │ ││ │││└─┴─┘│ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │││└───────┴─┴─────────────────┘│ │ ││ │││ │ ││ │ ││ ││└─────┴─┘│ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││└─────────────────────────────┴──┴─┘│ │││ │ ││ │ ││ │└─────────┴─┘│ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │└────────────────────────────────────┴─┘││ │ ││ │ │└─┴─────────────┴─┘│││││││ │
│ ││ │ ││ │ ││ │ ││ │ ││ │ │└─┴─┴───────────────────┘││││││ │
│ ││ │ ││ │ ││ │ ││ │ │└─┴─┴─────────────────────────┘│││││ │
│ ││ │ ││ │ ││ │ │└─┴────────────────────────────────────────┴───────────────────────────────┘││││ │
│ ││ │ ││ │ │└──┴──┴────────────────────────────────────────────────────────────────────────────┘│││ │
│ ││ │ │└─┴──┴────────────────────────────────────────────────────────────────────────────────────┘││ │
│ │└─────────┴─┴───────────────────────────────────────────────────────────────────────────────────────────┘│ │
└──┴─────────────────────────────────────────────────────────────────────────────────────────────────────────┴───────┘
add a comment |
up vote
1
down vote
C++, 202 190 188 bytes
int f(int*a,int l){int r=a[l-1]*10,i=0,j,k;for(;i<l;i++){for(j=l-1;j>i;j--){int s=1;for(k=i;k<j;k++)if(a[k+1]-a[k]<2)s=0;if(s&&j-i>1&&j!=l-1)r+=(a[j]-a[i])*(a[j]-a[i]),i=j,j=l;}}return r;}
Try it online!
Input must begin with 0.
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
5
down vote
Japt, 28 27 24 bytes
-1 byte from @kamil <3, -3 from @Shaggy :o. These guys are genius
ä- ô>2n)¯J lÉ mx x²+A*Uo
Try it online! or Test it all
Japt, 28 bytes, prev approach
Naive Way.
Important Input should start with 0 to work correctly (in some cases)
o *A+UóÈĦY©Y>XÃl>2 ®ä- xÃxp
Try it online! or Test it all
1
27 bytes
– Kamil Drakari
Nov 13 at 21:31
1
Quick 3 byte saving
– Shaggy
Nov 14 at 0:04
add a comment |
up vote
5
down vote
Japt, 28 27 24 bytes
-1 byte from @kamil <3, -3 from @Shaggy :o. These guys are genius
ä- ô>2n)¯J lÉ mx x²+A*Uo
Try it online! or Test it all
Japt, 28 bytes, prev approach
Naive Way.
Important Input should start with 0 to work correctly (in some cases)
o *A+UóÈĦY©Y>XÃl>2 ®ä- xÃxp
Try it online! or Test it all
1
27 bytes
– Kamil Drakari
Nov 13 at 21:31
1
Quick 3 byte saving
– Shaggy
Nov 14 at 0:04
add a comment |
up vote
5
down vote
up vote
5
down vote
Japt, 28 27 24 bytes
-1 byte from @kamil <3, -3 from @Shaggy :o. These guys are genius
ä- ô>2n)¯J lÉ mx x²+A*Uo
Try it online! or Test it all
Japt, 28 bytes, prev approach
Naive Way.
Important Input should start with 0 to work correctly (in some cases)
o *A+UóÈĦY©Y>XÃl>2 ®ä- xÃxp
Try it online! or Test it all
Japt, 28 27 24 bytes
-1 byte from @kamil <3, -3 from @Shaggy :o. These guys are genius
ä- ô>2n)¯J lÉ mx x²+A*Uo
Try it online! or Test it all
Japt, 28 bytes, prev approach
Naive Way.
Important Input should start with 0 to work correctly (in some cases)
o *A+UóÈĦY©Y>XÃl>2 ®ä- xÃxp
Try it online! or Test it all
edited Nov 14 at 2:03
answered Nov 13 at 19:29


Luis felipe De jesus Munoz
3,99421253
3,99421253
1
27 bytes
– Kamil Drakari
Nov 13 at 21:31
1
Quick 3 byte saving
– Shaggy
Nov 14 at 0:04
add a comment |
1
27 bytes
– Kamil Drakari
Nov 13 at 21:31
1
Quick 3 byte saving
– Shaggy
Nov 14 at 0:04
1
1
27 bytes
– Kamil Drakari
Nov 13 at 21:31
27 bytes
– Kamil Drakari
Nov 13 at 21:31
1
1
Quick 3 byte saving
– Shaggy
Nov 14 at 0:04
Quick 3 byte saving
– Shaggy
Nov 14 at 0:04
add a comment |
up vote
2
down vote
JavaScript (ES7), 72 bytes
a=>a.map(v=>(v-p>1?r=c++?r:p:t+=(c>1)*(c=0,p-r)**2,p=v),t=c=p=0)&&t+p*10
Try it online!
Commented
a => // a = input array
a.map(v => ( // for each value v in a:
v - p > 1 ? // if the difference with the previous floor is greater than 1:
r = c++ ? // increment the combo counter c; if c was not equal to 0:
r // leave r unchanged
: // else:
p // set r (reference floor) to the previous floor p
: // else:
t += // update t only ...
(c > 1) * ( // ... if we have a multi-floor jump combo of at least 2 jumps
c = 0, // reset c to 0
p - r // compute the total number of floors jumped in the combo
) ** 2, // square it and add it to t
p = v // set the previous value p to v
), //
t = c = p = 0 // start with t = c = p = 0
) && // end of map()
t + p * 10 // add the final floor multiplied by 10 to t
add a comment |
up vote
2
down vote
JavaScript (ES7), 72 bytes
a=>a.map(v=>(v-p>1?r=c++?r:p:t+=(c>1)*(c=0,p-r)**2,p=v),t=c=p=0)&&t+p*10
Try it online!
Commented
a => // a = input array
a.map(v => ( // for each value v in a:
v - p > 1 ? // if the difference with the previous floor is greater than 1:
r = c++ ? // increment the combo counter c; if c was not equal to 0:
r // leave r unchanged
: // else:
p // set r (reference floor) to the previous floor p
: // else:
t += // update t only ...
(c > 1) * ( // ... if we have a multi-floor jump combo of at least 2 jumps
c = 0, // reset c to 0
p - r // compute the total number of floors jumped in the combo
) ** 2, // square it and add it to t
p = v // set the previous value p to v
), //
t = c = p = 0 // start with t = c = p = 0
) && // end of map()
t + p * 10 // add the final floor multiplied by 10 to t
add a comment |
up vote
2
down vote
up vote
2
down vote
JavaScript (ES7), 72 bytes
a=>a.map(v=>(v-p>1?r=c++?r:p:t+=(c>1)*(c=0,p-r)**2,p=v),t=c=p=0)&&t+p*10
Try it online!
Commented
a => // a = input array
a.map(v => ( // for each value v in a:
v - p > 1 ? // if the difference with the previous floor is greater than 1:
r = c++ ? // increment the combo counter c; if c was not equal to 0:
r // leave r unchanged
: // else:
p // set r (reference floor) to the previous floor p
: // else:
t += // update t only ...
(c > 1) * ( // ... if we have a multi-floor jump combo of at least 2 jumps
c = 0, // reset c to 0
p - r // compute the total number of floors jumped in the combo
) ** 2, // square it and add it to t
p = v // set the previous value p to v
), //
t = c = p = 0 // start with t = c = p = 0
) && // end of map()
t + p * 10 // add the final floor multiplied by 10 to t
JavaScript (ES7), 72 bytes
a=>a.map(v=>(v-p>1?r=c++?r:p:t+=(c>1)*(c=0,p-r)**2,p=v),t=c=p=0)&&t+p*10
Try it online!
Commented
a => // a = input array
a.map(v => ( // for each value v in a:
v - p > 1 ? // if the difference with the previous floor is greater than 1:
r = c++ ? // increment the combo counter c; if c was not equal to 0:
r // leave r unchanged
: // else:
p // set r (reference floor) to the previous floor p
: // else:
t += // update t only ...
(c > 1) * ( // ... if we have a multi-floor jump combo of at least 2 jumps
c = 0, // reset c to 0
p - r // compute the total number of floors jumped in the combo
) ** 2, // square it and add it to t
p = v // set the previous value p to v
), //
t = c = p = 0 // start with t = c = p = 0
) && // end of map()
t + p * 10 // add the final floor multiplied by 10 to t
edited Nov 13 at 22:53
answered Nov 13 at 22:20


Arnauld
69.2k585293
69.2k585293
add a comment |
add a comment |
up vote
2
down vote
J, 54 bytes
[:((10*{:)+1#.[:}:]((2<#)*[:*:{:-{.);.1~1,2>2-~/])0&,
Try it online!
May add explanation later. For now here's an ungolfed and a parsed version:
ungolfed
[: ((10 * {:) + 1 #. [: }: ] ((2 < #) * [: *: {: - {.);.1~ 1 , 2 > 2 -~/ ]) 0&,
parsed
┌──┬─────────────────────────────────────────────────────────────────────────────────────────────────────────┬───────┐
│[:│┌─────────┬─┬───────────────────────────────────────────────────────────────────────────────────────────┐│┌─┬─┬─┐│
│ ││┌──┬─┬──┐│+│┌─┬──┬────────────────────────────────────────────────────────────────────────────────────┐│││0│&│,││
│ │││10│*│{:││ ││1│#.│┌──┬──┬────────────────────────────────────────────────────────────────────────────┐│││└─┴─┴─┘│
│ ││└──┴─┴──┘│ ││ │ ││[:│}:│┌─┬────────────────────────────────────────┬───────────────────────────────┐││││ │
│ ││ │ ││ │ ││ │ ││]│┌────────────────────────────────────┬─┐│┌─┬─┬─────────────────────────┐│││││ │
│ ││ │ ││ │ ││ │ ││ ││┌─────────────────────────────┬──┬─┐│~│││1│,│┌─┬─┬───────────────────┐││││││ │
│ ││ │ ││ │ ││ │ ││ │││┌───────┬─┬─────────────────┐│;.│1││ │││ │ ││2│>│┌─┬─────────────┬─┐│││││││ │
│ ││ │ ││ │ ││ │ ││ ││││┌─┬─┬─┐│*│┌──┬──┬─────────┐││ │ ││ │││ │ ││ │ ││2│┌─────────┬─┐│]││││││││ │
│ ││ │ ││ │ ││ │ ││ │││││2│<│#││ ││[:│*:│┌──┬─┬──┐│││ │ ││ │││ │ ││ │ ││ ││┌─────┬─┐│││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││└─┴─┴─┘│ ││ │ ││{:│-│{.││││ │ ││ │││ │ ││ │ ││ │││┌─┬─┐│/││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ ││ │ │└──┴─┴──┘│││ │ ││ │││ │ ││ │ ││ ││││-│~││ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ │└──┴──┴─────────┘││ │ ││ │││ │ ││ │ ││ │││└─┴─┘│ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │││└───────┴─┴─────────────────┘│ │ ││ │││ │ ││ │ ││ ││└─────┴─┘│ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││└─────────────────────────────┴──┴─┘│ │││ │ ││ │ ││ │└─────────┴─┘│ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │└────────────────────────────────────┴─┘││ │ ││ │ │└─┴─────────────┴─┘│││││││ │
│ ││ │ ││ │ ││ │ ││ │ ││ │ │└─┴─┴───────────────────┘││││││ │
│ ││ │ ││ │ ││ │ ││ │ │└─┴─┴─────────────────────────┘│││││ │
│ ││ │ ││ │ ││ │ │└─┴────────────────────────────────────────┴───────────────────────────────┘││││ │
│ ││ │ ││ │ │└──┴──┴────────────────────────────────────────────────────────────────────────────┘│││ │
│ ││ │ │└─┴──┴────────────────────────────────────────────────────────────────────────────────────┘││ │
│ │└─────────┴─┴───────────────────────────────────────────────────────────────────────────────────────────┘│ │
└──┴─────────────────────────────────────────────────────────────────────────────────────────────────────────┴───────┘
add a comment |
up vote
2
down vote
J, 54 bytes
[:((10*{:)+1#.[:}:]((2<#)*[:*:{:-{.);.1~1,2>2-~/])0&,
Try it online!
May add explanation later. For now here's an ungolfed and a parsed version:
ungolfed
[: ((10 * {:) + 1 #. [: }: ] ((2 < #) * [: *: {: - {.);.1~ 1 , 2 > 2 -~/ ]) 0&,
parsed
┌──┬─────────────────────────────────────────────────────────────────────────────────────────────────────────┬───────┐
│[:│┌─────────┬─┬───────────────────────────────────────────────────────────────────────────────────────────┐│┌─┬─┬─┐│
│ ││┌──┬─┬──┐│+│┌─┬──┬────────────────────────────────────────────────────────────────────────────────────┐│││0│&│,││
│ │││10│*│{:││ ││1│#.│┌──┬──┬────────────────────────────────────────────────────────────────────────────┐│││└─┴─┴─┘│
│ ││└──┴─┴──┘│ ││ │ ││[:│}:│┌─┬────────────────────────────────────────┬───────────────────────────────┐││││ │
│ ││ │ ││ │ ││ │ ││]│┌────────────────────────────────────┬─┐│┌─┬─┬─────────────────────────┐│││││ │
│ ││ │ ││ │ ││ │ ││ ││┌─────────────────────────────┬──┬─┐│~│││1│,│┌─┬─┬───────────────────┐││││││ │
│ ││ │ ││ │ ││ │ ││ │││┌───────┬─┬─────────────────┐│;.│1││ │││ │ ││2│>│┌─┬─────────────┬─┐│││││││ │
│ ││ │ ││ │ ││ │ ││ ││││┌─┬─┬─┐│*│┌──┬──┬─────────┐││ │ ││ │││ │ ││ │ ││2│┌─────────┬─┐│]││││││││ │
│ ││ │ ││ │ ││ │ ││ │││││2│<│#││ ││[:│*:│┌──┬─┬──┐│││ │ ││ │││ │ ││ │ ││ ││┌─────┬─┐│││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││└─┴─┴─┘│ ││ │ ││{:│-│{.││││ │ ││ │││ │ ││ │ ││ │││┌─┬─┐│/││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ ││ │ │└──┴─┴──┘│││ │ ││ │││ │ ││ │ ││ ││││-│~││ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ │└──┴──┴─────────┘││ │ ││ │││ │ ││ │ ││ │││└─┴─┘│ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │││└───────┴─┴─────────────────┘│ │ ││ │││ │ ││ │ ││ ││└─────┴─┘│ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││└─────────────────────────────┴──┴─┘│ │││ │ ││ │ ││ │└─────────┴─┘│ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │└────────────────────────────────────┴─┘││ │ ││ │ │└─┴─────────────┴─┘│││││││ │
│ ││ │ ││ │ ││ │ ││ │ ││ │ │└─┴─┴───────────────────┘││││││ │
│ ││ │ ││ │ ││ │ ││ │ │└─┴─┴─────────────────────────┘│││││ │
│ ││ │ ││ │ ││ │ │└─┴────────────────────────────────────────┴───────────────────────────────┘││││ │
│ ││ │ ││ │ │└──┴──┴────────────────────────────────────────────────────────────────────────────┘│││ │
│ ││ │ │└─┴──┴────────────────────────────────────────────────────────────────────────────────────┘││ │
│ │└─────────┴─┴───────────────────────────────────────────────────────────────────────────────────────────┘│ │
└──┴─────────────────────────────────────────────────────────────────────────────────────────────────────────┴───────┘
add a comment |
up vote
2
down vote
up vote
2
down vote
J, 54 bytes
[:((10*{:)+1#.[:}:]((2<#)*[:*:{:-{.);.1~1,2>2-~/])0&,
Try it online!
May add explanation later. For now here's an ungolfed and a parsed version:
ungolfed
[: ((10 * {:) + 1 #. [: }: ] ((2 < #) * [: *: {: - {.);.1~ 1 , 2 > 2 -~/ ]) 0&,
parsed
┌──┬─────────────────────────────────────────────────────────────────────────────────────────────────────────┬───────┐
│[:│┌─────────┬─┬───────────────────────────────────────────────────────────────────────────────────────────┐│┌─┬─┬─┐│
│ ││┌──┬─┬──┐│+│┌─┬──┬────────────────────────────────────────────────────────────────────────────────────┐│││0│&│,││
│ │││10│*│{:││ ││1│#.│┌──┬──┬────────────────────────────────────────────────────────────────────────────┐│││└─┴─┴─┘│
│ ││└──┴─┴──┘│ ││ │ ││[:│}:│┌─┬────────────────────────────────────────┬───────────────────────────────┐││││ │
│ ││ │ ││ │ ││ │ ││]│┌────────────────────────────────────┬─┐│┌─┬─┬─────────────────────────┐│││││ │
│ ││ │ ││ │ ││ │ ││ ││┌─────────────────────────────┬──┬─┐│~│││1│,│┌─┬─┬───────────────────┐││││││ │
│ ││ │ ││ │ ││ │ ││ │││┌───────┬─┬─────────────────┐│;.│1││ │││ │ ││2│>│┌─┬─────────────┬─┐│││││││ │
│ ││ │ ││ │ ││ │ ││ ││││┌─┬─┬─┐│*│┌──┬──┬─────────┐││ │ ││ │││ │ ││ │ ││2│┌─────────┬─┐│]││││││││ │
│ ││ │ ││ │ ││ │ ││ │││││2│<│#││ ││[:│*:│┌──┬─┬──┐│││ │ ││ │││ │ ││ │ ││ ││┌─────┬─┐│││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││└─┴─┴─┘│ ││ │ ││{:│-│{.││││ │ ││ │││ │ ││ │ ││ │││┌─┬─┐│/││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ ││ │ │└──┴─┴──┘│││ │ ││ │││ │ ││ │ ││ ││││-│~││ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ │└──┴──┴─────────┘││ │ ││ │││ │ ││ │ ││ │││└─┴─┘│ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │││└───────┴─┴─────────────────┘│ │ ││ │││ │ ││ │ ││ ││└─────┴─┘│ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││└─────────────────────────────┴──┴─┘│ │││ │ ││ │ ││ │└─────────┴─┘│ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │└────────────────────────────────────┴─┘││ │ ││ │ │└─┴─────────────┴─┘│││││││ │
│ ││ │ ││ │ ││ │ ││ │ ││ │ │└─┴─┴───────────────────┘││││││ │
│ ││ │ ││ │ ││ │ ││ │ │└─┴─┴─────────────────────────┘│││││ │
│ ││ │ ││ │ ││ │ │└─┴────────────────────────────────────────┴───────────────────────────────┘││││ │
│ ││ │ ││ │ │└──┴──┴────────────────────────────────────────────────────────────────────────────┘│││ │
│ ││ │ │└─┴──┴────────────────────────────────────────────────────────────────────────────────────┘││ │
│ │└─────────┴─┴───────────────────────────────────────────────────────────────────────────────────────────┘│ │
└──┴─────────────────────────────────────────────────────────────────────────────────────────────────────────┴───────┘
J, 54 bytes
[:((10*{:)+1#.[:}:]((2<#)*[:*:{:-{.);.1~1,2>2-~/])0&,
Try it online!
May add explanation later. For now here's an ungolfed and a parsed version:
ungolfed
[: ((10 * {:) + 1 #. [: }: ] ((2 < #) * [: *: {: - {.);.1~ 1 , 2 > 2 -~/ ]) 0&,
parsed
┌──┬─────────────────────────────────────────────────────────────────────────────────────────────────────────┬───────┐
│[:│┌─────────┬─┬───────────────────────────────────────────────────────────────────────────────────────────┐│┌─┬─┬─┐│
│ ││┌──┬─┬──┐│+│┌─┬──┬────────────────────────────────────────────────────────────────────────────────────┐│││0│&│,││
│ │││10│*│{:││ ││1│#.│┌──┬──┬────────────────────────────────────────────────────────────────────────────┐│││└─┴─┴─┘│
│ ││└──┴─┴──┘│ ││ │ ││[:│}:│┌─┬────────────────────────────────────────┬───────────────────────────────┐││││ │
│ ││ │ ││ │ ││ │ ││]│┌────────────────────────────────────┬─┐│┌─┬─┬─────────────────────────┐│││││ │
│ ││ │ ││ │ ││ │ ││ ││┌─────────────────────────────┬──┬─┐│~│││1│,│┌─┬─┬───────────────────┐││││││ │
│ ││ │ ││ │ ││ │ ││ │││┌───────┬─┬─────────────────┐│;.│1││ │││ │ ││2│>│┌─┬─────────────┬─┐│││││││ │
│ ││ │ ││ │ ││ │ ││ ││││┌─┬─┬─┐│*│┌──┬──┬─────────┐││ │ ││ │││ │ ││ │ ││2│┌─────────┬─┐│]││││││││ │
│ ││ │ ││ │ ││ │ ││ │││││2│<│#││ ││[:│*:│┌──┬─┬──┐│││ │ ││ │││ │ ││ │ ││ ││┌─────┬─┐│││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││└─┴─┴─┘│ ││ │ ││{:│-│{.││││ │ ││ │││ │ ││ │ ││ │││┌─┬─┐│/││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ ││ │ │└──┴─┴──┘│││ │ ││ │││ │ ││ │ ││ ││││-│~││ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││││ │ │└──┴──┴─────────┘││ │ ││ │││ │ ││ │ ││ │││└─┴─┘│ ││ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │││└───────┴─┴─────────────────┘│ │ ││ │││ │ ││ │ ││ ││└─────┴─┘│ ││ ││││││││ │
│ ││ │ ││ │ ││ │ ││ ││└─────────────────────────────┴──┴─┘│ │││ │ ││ │ ││ │└─────────┴─┘│ ││││││││ │
│ ││ │ ││ │ ││ │ ││ │└────────────────────────────────────┴─┘││ │ ││ │ │└─┴─────────────┴─┘│││││││ │
│ ││ │ ││ │ ││ │ ││ │ ││ │ │└─┴─┴───────────────────┘││││││ │
│ ││ │ ││ │ ││ │ ││ │ │└─┴─┴─────────────────────────┘│││││ │
│ ││ │ ││ │ ││ │ │└─┴────────────────────────────────────────┴───────────────────────────────┘││││ │
│ ││ │ ││ │ │└──┴──┴────────────────────────────────────────────────────────────────────────────┘│││ │
│ ││ │ │└─┴──┴────────────────────────────────────────────────────────────────────────────────────┘││ │
│ │└─────────┴─┴───────────────────────────────────────────────────────────────────────────────────────────┘│ │
└──┴─────────────────────────────────────────────────────────────────────────────────────────────────────────┴───────┘
answered Nov 14 at 4:45


Jonah
1,951816
1,951816
add a comment |
add a comment |
up vote
1
down vote
C++, 202 190 188 bytes
int f(int*a,int l){int r=a[l-1]*10,i=0,j,k;for(;i<l;i++){for(j=l-1;j>i;j--){int s=1;for(k=i;k<j;k++)if(a[k+1]-a[k]<2)s=0;if(s&&j-i>1&&j!=l-1)r+=(a[j]-a[i])*(a[j]-a[i]),i=j,j=l;}}return r;}
Try it online!
Input must begin with 0.
add a comment |
up vote
1
down vote
C++, 202 190 188 bytes
int f(int*a,int l){int r=a[l-1]*10,i=0,j,k;for(;i<l;i++){for(j=l-1;j>i;j--){int s=1;for(k=i;k<j;k++)if(a[k+1]-a[k]<2)s=0;if(s&&j-i>1&&j!=l-1)r+=(a[j]-a[i])*(a[j]-a[i]),i=j,j=l;}}return r;}
Try it online!
Input must begin with 0.
add a comment |
up vote
1
down vote
up vote
1
down vote
C++, 202 190 188 bytes
int f(int*a,int l){int r=a[l-1]*10,i=0,j,k;for(;i<l;i++){for(j=l-1;j>i;j--){int s=1;for(k=i;k<j;k++)if(a[k+1]-a[k]<2)s=0;if(s&&j-i>1&&j!=l-1)r+=(a[j]-a[i])*(a[j]-a[i]),i=j,j=l;}}return r;}
Try it online!
Input must begin with 0.
C++, 202 190 188 bytes
int f(int*a,int l){int r=a[l-1]*10,i=0,j,k;for(;i<l;i++){for(j=l-1;j>i;j--){int s=1;for(k=i;k<j;k++)if(a[k+1]-a[k]<2)s=0;if(s&&j-i>1&&j!=l-1)r+=(a[j]-a[i])*(a[j]-a[i]),i=j,j=l;}}return r;}
Try it online!
Input must begin with 0.
edited Nov 14 at 12:57
answered Nov 13 at 22:23
Zacharý
5,10511035
5,10511035
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f175875%2fcalculate-your-icy-tower-score%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Map6k3xbuJPsx JvOt8d2,4BB788TOmd BtZxr3NK68FJ0mJGnut2oCRwz,kKteBEoTfunwH6y5,YWDO